Using Python for GET API Calls: A Step-by-Step Guide for Developers
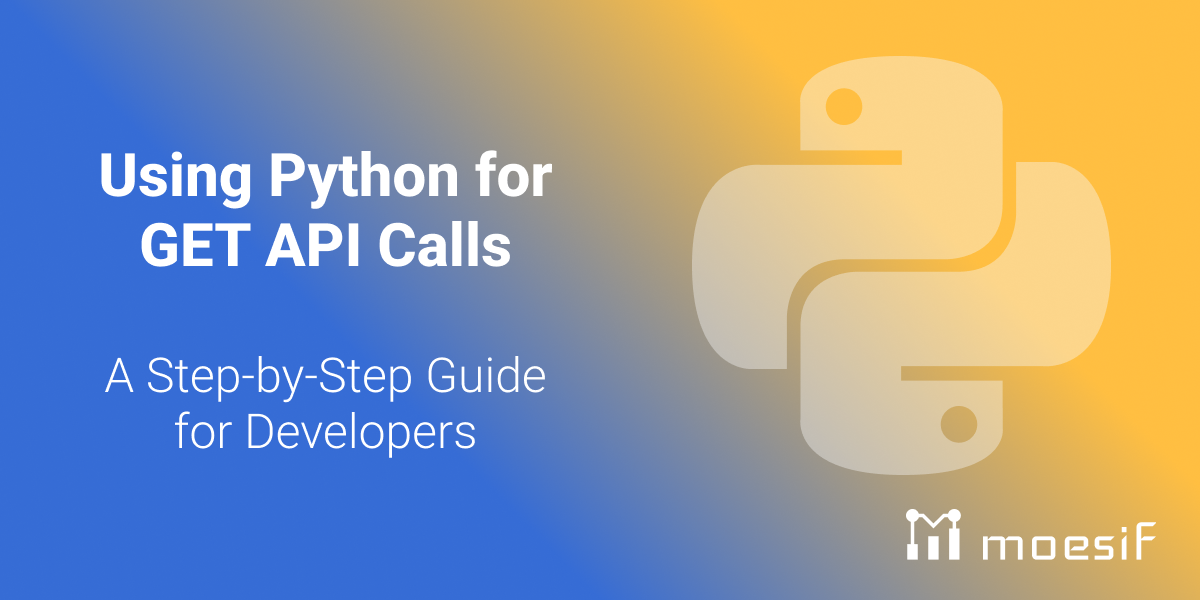
Understanding how to make a GET request to an API using Python is an essential skill for developers. This article will guide you through the process, demonstrating how to use Python’s ‘requests’ library to fetch data, handle the full JSON object in response, and manage API errors efficiently. Step into the practical world of Python GET API calls without any detours.
Key Takeaways
-
Python is an ideal language for API consumption and development due to its straightforward syntax and extensive library ecosystem, making API interactions simpler and more efficient.
-
The Python ‘requests’ library is a fundamental tool for API interaction, enabling easy installation, seamless HTTP requests, and the handlings of responses, including parsing JSON data.
-
Understanding and using HTTP status codes is critical for handling API requests and responses effectively; mastering API documentation is essential for effective API use, and hands-on examples like fetching weather data and accessing social media APIs demonstrate Python’s versatility.
Understanding APIs and Their Importance
APIs, or Application Programming Interfaces, serve as a system that bridges the gap between different software applications, enabling a standardized method for data exchange and interaction. Imagine a world where applications could communicate seamlessly, where data could be exchanged without hassles, and where software ecosystems could interact efficiently. That’s the world APIs help create!
As a language, Python has found its niche in API consumption and development, thanks to its straightforward syntax and extensive library ecosystem. It serves as a universal translator in the realm of APIs, effortlessly simplifying complex procedures and interactions.
API Types and Protocols
Among the various types of APIs, REST APIs, which use HTTP for data exchange, are the most popular and flexible. The REST architecture simplifies client/server communication access data together, making data access more straightforward and logical, akin to how a well-organized library simplifies the search for a particular book. By utilizing a REST API, developers can easily integrate various systems and applications, enhancing their functionality and user experience.
APIs can be categorized based on architecture, such as SOAP or REST, and by their intended scope of use, like private, public, partner, and composite APIs. It’s like having different routes to reach a destination, each with its unique advantages and challenges.
The Role of Python in API Development
The simplicity of Python’s syntax and its comprehensive library ecosystem make it an attractive choice for developers. It equates to a powerful tool that facilitates tasks effortlessly.
Python’s capability is boosted by frameworks such as Django and Flask that aid API development, allowing developers to construct robust API suites with speed and efficiency using python code. This could be compared to owning a power drill that not only drills holes rapidly but also provides a wide range of other functions, much like a versatile python object.
Setting Up Your Python Environment for API Requests
Whether you’re a Windows, Mac OS X, or Linux user, setting up your Python environment for API requests is a straightforward process. It’s as simple as downloading and running the Python installer, adding Python to the PATH, and installing the requests package via the command line.
The requests library is a common choice for API interaction in Python. To start using it, simply import requests. Think of it as your passport for a smooth and efficient journey through the world of APIs.
Installing the Requests Library
The Python Requests library is an essential tool in a developer’s arsenal, simplifying the process of making HTTP requests to APIs and web services. Installing it is as easy as using the package manager pip, which works across various operating systems, including Windows, Mac OS X, and Linux.
By using the simple console command ‘pip install requests’, you can install the Requests library and initiate your exploration of API interactions. Consider it as pressing the ‘Start’ button on your journey towards mastering APIs.
Importing Required Libraries
Make sure to equip yourself with the necessary tools before embarking on your journey. In the realm of Python and APIs, these tools are the libraries you need to import into your Python script.
The ‘requests’ library comes recommended for consuming APIs with Python. Once installed, it must be imported into your code to be utilized, much like starting your car’s engine before you set off on a journey.
Making Your First API Request with Python
To make a GET request in Python, you typically use the ‘requests.get()’ method in conjunction with the request library or API’s URL endpoint. It is comparable to dialing a phone number to make a call. The Python Requests library, which simplifies HTTP requests, is frequently used to interact with REST APIs.
An endpoint in the context of APIs is a specific URL that specifies the exact requested resource, or data that a developer wishes to retrieve through an API call. By using an API endpoint, API responses include headers and the requested data or payload, often formatted as JSON, making it essential for developers to understand how to handle this format.
Analyzing API Responses
Upon making a GET request to api server to retrieve data, the server offers post request a response object carrying status codes and response data that indicate whether the request was successful or not. This is similar to receiving a delivery confirmation text after ordering a pizza, as it helps in managing response requests.
To extract the valuable content from the API response, developers can employ various methods like ‘response.content()’, ‘response.text()’, or ‘response.json()’, the latter being specifically designed for JSON-formatted data. HTTP headers in the request data response can provide extra parameters that govern the exchange data request and response, offering valuable information for developers.
Parsing JSON Data
JSON, the language of APIs, is a lightweight data-interchange format that is machine-readable and favored for its simplicity, flexibility, and ease of use for humans. It’s like the English language in the world of APIs, widely understood and easy to use. the JSON format, short for JavaScript Object Notation, has become the go-to choice for developers when working with APIs.
With Python’s built-in json module, encoding and decoding JSON and data formats becomes simpler, easing the interaction between Python programs and JSON-formatted API data. To readily access and manipulate the JSON data from an API response, the ‘response.json()’ method is used, which converts the whole JSON string of data into a Python dictionary. This is similar to translating text from a foreign language into your native tongue for better comprehension.
Working with Query Parameters in Python
Query parameters are the secret sauce in your API requests. They are filters that can be sent with an API request to narrow down the responses and refine data requests according required parameters or to specific requirements, analogous to ordering a customized burger with specific instructions.
Query parameters empower developers to filter results according to specific criteria. This customizes the behavior of AI models and provides selective access to relevant data subsets when making API requests. It can be compared to using a specific keyword to find relevant results on a search engine.
Constructing API Requests with Query Parameters
Adding query parameters to API requests can be done using the ‘params’ argument in ‘requests.get()’, which accepts a dictionary of parameters.
When constructing API requests with multiple query parameters, in Python, a dictionary can be used to hold varying key-value pairs that represent all the data query parameters. It’s like packing a suitcase with different items for a trip, each item representing a query parameter.
Best Practices for Using Query Parameters
Just like there are best practices for writing efficient code for asynchronous requests, there are also best practices for using query parameters in API requests. Understanding these practices can help you make the most of your API interactions.
Whether it’s understanding the API documentation, using the ‘params’ attribute, or constructing advanced requests with lists of items, these practices will guide you in crafting effective and simple API requests. It’s like learning the rules of a game to play it well.
Handling API Errors and Status Codes
As with any journey, the journey through APIs can come with its share of hiccups. Status codes, part of the HTTP protocol, indicate the result of a request, communicating the outcome of API requests such as success, failure, or error types.
Comprehending status codes is vital since they guide applications on how to manage responses, indicating success, failure, or the requirement for further action, such as providing missing data. Understanding the meaning of each status code is akin to road signs that aid in your journey, steering you efficiently.
Common API Status Codes
HTTP status codes categorized under 2xx indicate successful requests, while 4xx codes indicate client errors and 5xx codes indicate server errors. It’s like traffic lights, each color and status code indicating a different action to be taken.
A Response [200] means that everything went okay, indicating that an API request was successful.
Implementing Error Handling in Python
To implement error handling in Python, you can follow these steps:
-
Use the ‘response.raise_for_status()’ method to check for any HTTP errors.
-
Catch exceptions such as HTTPError, ConnectionError, and Timeout to handle different types of errors.
-
Handle the errors appropriately, such as displaying an error message or retrying the request.
Implementing error handling in your code is like carrying a first-aid kit during a journey, preparing you for any unexpected situations.
To handle API errors, use try-except blocks to catch exceptions like:
- HTTPError
- ConnectionError
- Timeout
- RequestException
These exceptions cater to handling different types of request issues including exceeding rate limits. It’s like having different tools to fix different issues in a car, preparing you for any eventuality.
Navigating and Understanding API Documentation
Understanding API documentation provides guidelines on how to effectively use API services, incorporating crucial information about available endpoints http methods, and resources, and how to navigate them to construct requests and handle responses.
Understanding the documentation thoroughly involves obtaining an API key and integrating the API keys with python itself as per the documentation much like learning the rules and strategies of a game before starting to play.
Finding API Documentation
API reference documentation is typically found on the platform where the API is hosted, under a ‘Documentation’ tab.
While the access token visibility of API documentation might be restricted and require an invitation, many APIs have their documentation publicly available and searchable on the developer’s portal. It’s like open-source software, accessible to anyone who wishes to use it.
Reading and Interpreting API Documentation
Reading and interpreting API documentation can seem daunting at first, but with a little practice, it becomes a breeze. API documentation may include different sections such as an overview, a developer guide, and a user’s guide tailored to the audience.
Generated API documentation provides a top-level view of operations, and detailed views filtering data used for each operation, and enables developers to test the API by sending and receiving messages.
Practical Examples of Python API Requests
Python demonstrates its versatility in API interaction through diverse use cases, and following a Python API tutorial can help you explore these possibilities. These include fetching weather data and accessing social media data, showcasing the boundless possibilities for developers. This is similar to a Swiss army knife application programming interface, providing a tool for every task.
From interacting with the World Bank’s Development Indicators to fetching trending GIFs from the GIPHY website, Python’s Requests library makes these tasks effortless.
Fetching Weather Data
Fetching weather data is a common use case for APIs, and OpenWeatherMap provides weather data services that can be accessed with an API key.
To access current weather information from OpenWeatherMap’s API, the ‘requests’ module is used for the API call, while the ‘json’ module manages the json response itself. This is comparable to using a remote control to turn on your TV and tune into your preferred channel.
Accessing Social Media Data
In the age of social media, Python can be used to automate social media postings on platforms like Facebook, Twitter, and Instagram using their respective APIs.
Whether you’re posting text messages and images on Facebook using the Graph API or scheduling posts for automatic posting at specific times using Python’s ‘schedule’ library, the opportunities are limitless.
Effortless Python Integration with Moesif’s Powerful SDK
Moesif simplifies API analytics for Python developers. Our Python SDK streamlines integration, allowing you to effortlessly capture valuable API usage data directly within your Python applications. This empowers you to gain deep insights into developer behavior and optimize your APIs for maximum performance and user satisfaction.
Summary
As we wrap up this journey through the world of APIs and Python, it’s clear that APIs are crucial for communication between software applications, and Python is a popular language for API development and consumption. The versatility of Python in API interactions, from fetching weather data to automating social media postings, reveals the extensive possibilities for developers. With the right tools and understanding, you too can master the Python Get API and harness its potential to create powerful, data-driven applications.
Organizations looking for the best tools to support their REST API management can leverage Moesif’s powerful API analytics and monetization capabilities. Moesif easily integrates with your favorite API management platform or API gateway through one of our easy-to-use plugins, or embed Moesif directly into your API code using one of our SDKs. To try it yourself, sign up today and start with a 14-day free trial; no credit card is required.