Building a RESTful API with Rails
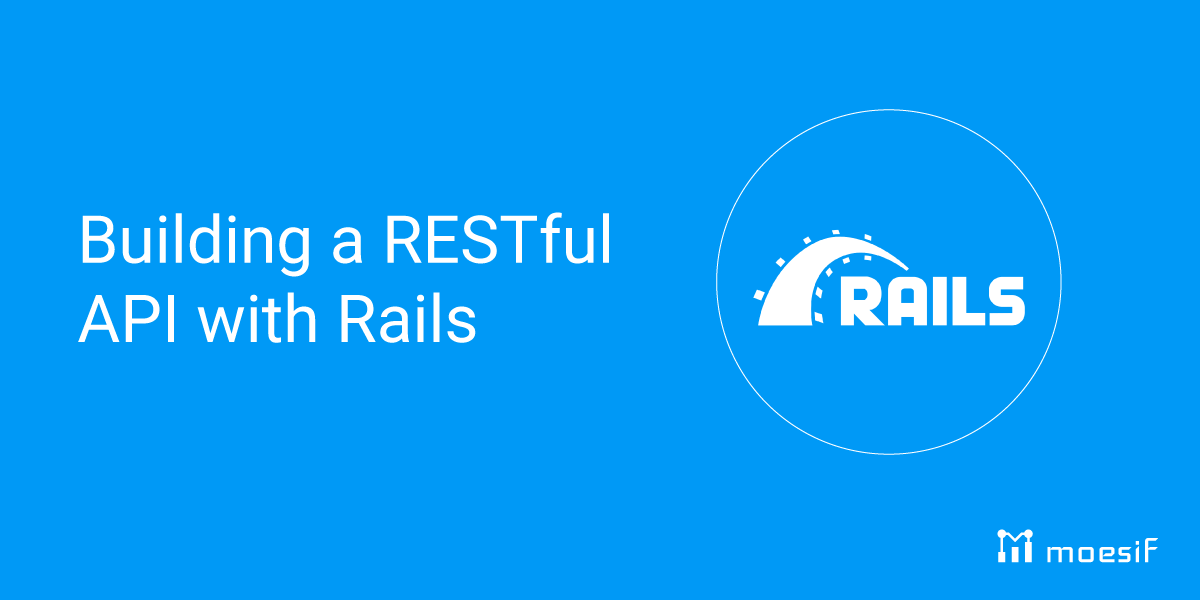
When it comes to building an API, Rails is an extremely popular programming language choice to build powerful RESTful APIs. In this tutorial, we will build a simple REST API using Rails. The Rails REST API endpoint will allow users to retrieve a credit score rating. Of course, we won’t be linking up to any backend systems to pull a credit score but will instead use a random number generator to generate the score and return it to the user. Although simple, this tutorial will show you the basics of REST API development with Rails.
Prerequisites
Before we start, we must ensure that a couple of prerequisites are completed. To follow along and run this tutorial, you will need to:
- Have Ruby installed
- Install Rails -
sudo gem install rails
- Have an IDE available to edit your code
- Install Postman so that you can test the endpoint
After all 4 of these prerequisites are completed, we can begin!
Adding in the Code
Our first step in creating our code is to generate a Rails API project. To do this, we will run the following command to create a new Rails API application.
rails new my_api --api
Optionally, we will also set up the CORS configuration to allow traffic from all origins to make things easier for testing. In the Gemfile in the root directory, uncomment the rack-cors
entry. It should then look like this.
gem "rack-cors"
Once this is uncommented, we need to install the dependency. Then, in your terminal, run the following to install the dependency.
bundle install
In the root folder of the project, in the config/initializers
directory, open the cors.rb file. In the cors.rb file, uncomment the CORS config, and change the origins
entry to “*”
. This will allow ALL origins, essentially allowing all traffic to flow into the service. For product applications, you’ll likely want to lock things down and dial in the CORS config a bit more.
The file should now look like this:
Rails.application.config.middleware.insert_before 0, Rack::Cors do
allow do
origins "*"
resource "*",
headers: :any,
methods: [:get, :post, :put, :patch, :delete, :options, :head]
end
end
Next, we will create our GET
endpoint for api/getcreditscore
. Our first step is to create the route for the endpoint. To do this, open the routes.rb file in the config
directory.
Rails.application.routes.draw do
# Define your application routes per the DSL in https://guides.rubyonrails.org/routing.html
# Defines the root path route ("/")
# root "articles#index"
get 'api/getcreditscore', to: 'application#creditscore'
end
Now that the route is defined, we will create the controller function for the endpoint. The function will return a JSON payload with a random number between 500 and 900, representing a credit score. To add this logic in, navigate to the application_controller.rb in the controllers
directory. The completed controller file will look like the example below.
class ApplicationController < ActionController::API
def creditscore
render json: { creditscore: rand(500..900) }
end
end
With that, our API is ready to be deployed.
Running and Testing the Code
In this case, we will run our Rails app locally. To run the API locally we will need to start up the Rails server. We can do this by using the rails server
command in the root directory of the app.
rails server
Now, our Rails application and API are up and running. You can send a test HTTP request through Postman. By sending a request to localhost:3000/api/getcreditscore
, you should see a 200 OK
status code and a credit score returned from the creditscore
handler written in the application_controller.rb file. For this test, no request body is needed for the incoming request.
By default, rails will run on port 3000. To change this, you can open the puma.rb file in the
config
directory and change the following line of code to patch the port you want to have the server listen on.
port ENV.fetch("PORT") { 3000 }
Wrapping Up
With that, we’ve created a simple RESTful API using Rails. This code can then be expanded on as needed to build APIs for your applications. Moving forward, you may want to secure the API with an API key, integrate the API with an API gateway, check out how your API is being consumed and used, or build revenue through API monetization. For a solution to your API analytics and monetization needs, check out Moesif today to explore all of this and more!