How to Use an API: A Step-by-Step Tutorial for Beginners
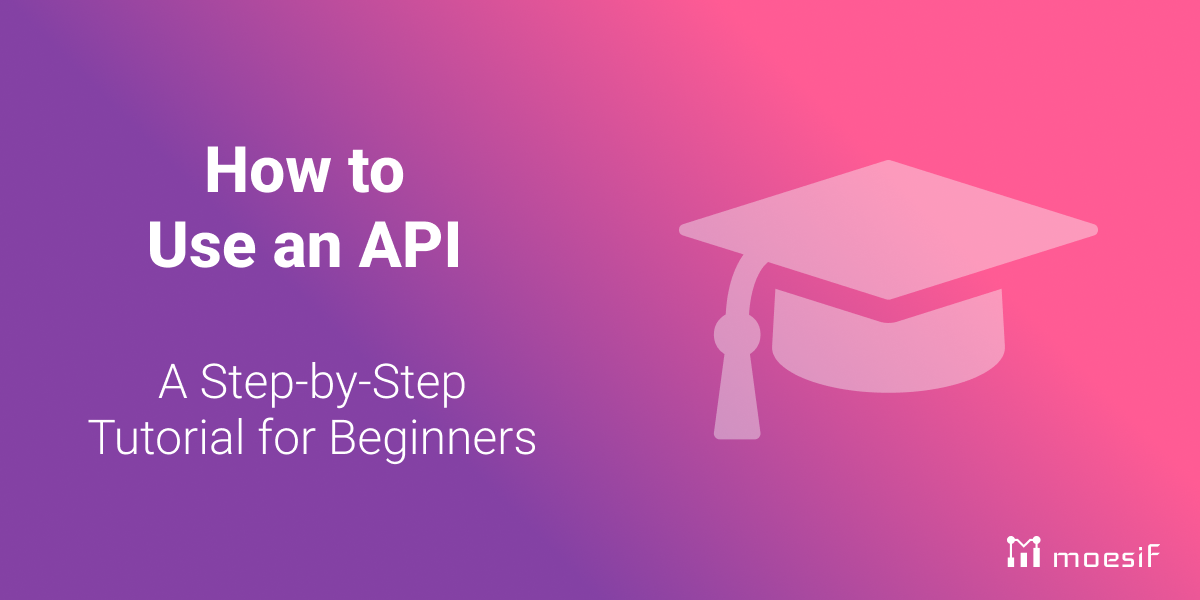
Understanding how to use an API is essential for modern software development, from simple data retrieval to complex integrations. This guide demystifies the process, providing you with the insights you need to make API requests, decode responses, and securely implement these powerful connectors in your code. Step by step, you’ll learn the key concepts that will empower you to leverage APIs effectively in your own projects.
Key Takeaways
- APIs (Application Programming Interfaces) are crucial for enabling communication between software applications, defining how requests are made, data formats used, and protocols to follow.
- There are various types of APIs such as REST, SOAP, GraphQL, and WebSocket, each with unique capabilities; choosing the right type depends on the specific needs of the application.
- Securing API access with API keys and authorization methods is essential, and developers must create, send, and handle API requests correctly while ensuring proper API integration and performance monitoring in their applications.
Understanding APIs: The Basics
Essentially, an API, short for Application Programming Interface, serves as a software intermediary, facilitating communication between two applications. Think of it as a messenger, transporting requests from one system to another, and ensuring the correct response is delivered. APIs have become a fundamental component of modern software development, powering everything from social media platforms to e-commerce transactions, all thanks to the power of application programming interfaces.
Essentially, APIs act as intermediaries to facilitate mutual understanding and cooperation between various software applications. They:
- Define the kinds of requests that can be made
- Specify how to make the requests
- Determine the data formats that should be used
- Establish the conventions to follow.
Ever wondered how your weather app updates with real-time data? Or how you can share a YouTube video on your Facebook page with just a click? That’s APIs at work, silently and efficiently connecting different software systems and enabling them to share data and functionalities.
Types of APIs: Exploring the Landscape
The API landscape is diverse, comprising several types, each boasting unique benefits and applications. Among them, REST (Representational State Transfer) APIs, also known as rest api, are one of the most popular. REST APIs operate based on the HTTP standard and can handle different data formats like XML, JSON, and HTML, making them a flexible and interoperable choice for developers.
On the other hand, there are different types of web APIs that serve different purposes:
SOAP APIs
SOAP (Simple Object Access Protocol) APIs are typically used in scenarios where formal contracts and long-running processes are involved. They are used for operations such as creating, retrieving, updating, or deleting records from a server.
GraphQL APIs
GraphQL APIs offer clients the ability to retrieve precise data tailored to their requirements, often using JSON as the data format.
WebSocket APIs
WebSocket APIs enable real-time communication and updates, proving indispensable in applications that require live interactions.
Whether it’s a REST, SOAP, GraphQL, or WebSocket API, each type brings unique capabilities to the table. The key is to understand your application’s needs and choose an API that best aligns with those requirements.
Acquiring API Keys and Authorization
API keys and authorization methods are indispensable in securing access to APIs and managing data usage. An API key is a unique sequence of characters used to authenticate and authorize users accessing an API, serving as a security measure to regulate API access and protect sensitive information. Obtaining an API key typically involves logging into a developer account on the API provider’s website, accessing the section for API keys, and requesting a new key.
API authorization, on the other hand, verifies the identity of the user or application initiating the API request, ensuring they have the necessary permissions to interact with the API and its resources. This is often accomplished using standards like OAuth, which allows a website or application to access resources from other web applications without revealing user credentials, providing a secure and regulated method for granting permissions and accessing protected resources.
API Documentation: A Developer’s Guide
API documentation serves as a comprehensive guide for developers, outlining essential information for effective API usage. It includes:
- A comprehensive description of the API, detailing its functionalities, constraints, and prerequisites
- Examples of each call, parameter, and response
- Code samples, references, tutorials, and descriptive explanations
To make the most of API documentation, it’s advisable to start by familiarizing oneself with API terminology, then delving into the API overview to understand its objectives and capabilities. The API reference provides detailed information about accessible resources and their interaction methods. Lastly, reviewing available tutorials can help gain hands-on knowledge on API utilization.
Creating Your First API Request
Prepared for your first API request? The procedure entails endpoint selection, parameter and header configuration, along with API response management. Let’s break it down into three steps.
Choosing an Endpoint
An API endpoint is essentially a specific URL that provides access to a resource on the server. It’s the point of communication between an API client and an API server, where requests are received and responses are sent. Each endpoint is comprised of:
- An HTTP method
- An endpoint URL
- Headers
- A body
This API provider offers an organized way to manage private APIs functionalities.
When selecting an endpoint, it’s important to consider factors such as:
- Documentation
- Libraries
- Consistency
- Support
- Reputation
- Pricing
- Data privacy
Additionally, understanding the difference between HTTP methods like POST and GET is crucial. While GET API endpoints are used for data retrieval, POST API endpoints are employed for data creation. GET requests are visible in the URL and are thus considered less secure, while POST requests are more secure as the data is transmitted in the request body and hidden from the URL.
Setting Parameters and Headers
Parameters and headers are key components of API requests, allowing you to customize your requests and provide additional information to the server. API request parameters are configurable options that you can include with the endpoint to affect the response, acting as filters for your search. You can set these parameters as key-value pairs appended to the URL or as header parameters included in the request headers.
Headers, on the other hand, provide supplementary meta-information to the server. This can include data about the request, authentication details, and more. To set headers in languages like JavaScript, methods like ‘setRequestHeader’ of an XMLHttpRequest object are used.
Understanding the function and implementation of both parameters and headers is essential for effective API usage.
Handling API Responses
Once you’ve made an API request, you’ll receive a response. Managing these responses is a crucial part of working with APIs. A key aspect of this is understanding status codes. HTTP response status codes indicate whether a specific HTTP request has been successfully completed, providing valuable information about the outcome of the API request.
API responses can be returned in various formats, including:
- JSON
- XML
- Plain Text
- Binary
Depending on your programming language, different methods can be used to parse this data, such as javascript object notation (JSON). For example, in Python, you can use the json module to parse JSON data from an API response. In Java, libraries like DOM or SAX parsers can be used to parse XML data.
Understanding how to handle and interpret API responses is key to successfully interacting with APIs.
Integrating APIs into Your Application
Now, equipped with knowledge of making API requests and managing responses, it’s time to explore choosing an appropriate API for your requirements and incorporating API calls into your application code.
Selecting the Right API for Your Needs
Choosing the right API for your application is a crucial decision that can impact your application’s functionality and performance. Your business requirements, budget, and the compatibility of the API with your existing application are all important factors to consider in this process. There are also cost-effective APIs suitable for small businesses such as Postman, Amazon API Gateway, and Stoplight, among others.
Testing and automation scripts can be used to evaluate the compatibility of an API with your existing application. When selecting an API for business purposes, it is also important to consider factors such as:
- Ease of use
- Scalability
- Security
- Flexibility
- Comprehensiveness
Implementing API Calls in Your Code
Once you’ve chosen the right API, the next step is to integrate API calls into your code. This involves:
- Selecting the right API
- Acquiring an API key if necessary
- Making an HTTP request to the API endpoint
- Receiving the response
- Processing the response within your code.
Depending on your application’s technology stack, you may use different libraries or tools to make these API calls. For example:
- In a Python application, you can use the requests library.
- In a Node.js application, libraries like Axios, node-fetch, or SuperAgent can be useful.
- Even in languages like PHP, making API calls is feasible using libraries like cURL or by developing a utility function.
It’s also important to follow best practices when implementing API calls in your code, such as using nouns for resource identification, ensuring proper HTTP headers, and implementing thorough error handling.
Securing and Monitoring Your API Usage
APIs, while powerful, necessitate responsible usage. The implementation of security measures is vital to safeguard your API usage, alongside performance monitoring to maximize efficiency and ward off unauthorized access.
Implementing Security Measures
Security is of utmost importance when using APIs. API keys and authentication tokens are key to protecting your API usage, and it’s crucial to store and manage these securely. OAuth2 is a widely used standard for API authorization, providing a secure method to grant permissions and access protected resources.
In addition to authentication and authorization, securing your API endpoints from abuse is also vital. This can be achieved by:
- Designing the API with a minimal exposure surface
- Following best practices for authentication and authorization
- Implementing rate limiting
- Regularly updating and patching the API to address security vulnerabilities.
JWT authentication is another method for securing API usage, where a token is generated upon user sign-in and used in subsequent API requests to authenticate and gain access to protected endpoints. This process ensures smooth API integration for users.
Monitoring API Performance
In addition to securing your API usage, monitoring API performance is critical to maintaining a high-quality user experience and identifying issues early. This can be done by:
- Tracking API availability
- Measuring response time
- Monitoring error rates
- Validating functional uptime
- Tracking API dependencies
There are a variety of tools available to monitor API performance across different technology stacks. Some of these tools include:
- Moesif: an analytics and billing platform designed to help businesses understand and monetize their API usage. It offers features for tracking customer interactions, setting up usage-based billing, and providing real-time alerts, aiming to support product-led enterprises and startups in growing their API products.
- AppMetrics: used for real-time monitoring and analysis in a Node.js application
- Opbeat: provides automated performance tracking for Django
- Sematext, Prometheus, Uptrends, AppDynamics, SigNoz, Datadog, and New Relic: effective tools that can help monitor API performance across different platforms.
Continuous monitoring of API performance is crucial to maintaining optimal API performance and providing the best possible user experience.
Real-Life API Examples and Use Cases
Chances are, you’ve engaged with APIs more frequently than you’re aware of. From checking the weather on your phone to streaming your favorite song on Spotify, APIs are everywhere. In fact, when you use an API like the Google Maps API, it allows you to access real-time experiences like plane tracking and displaying weather conditions on maps. Composite APIs can further enhance these experiences by combining multiple data sources and services.
Businesses also leverage APIs to enhance their services. The X (Twitter) API, for example, is used to organize work, manage API access, monitor data, interact with tweets and profiles, analyze social media information, and engage with an X conversation. The Spotify API, on the other hand, allows developers to build applications that interact with Spotify’s music streaming service, offering functionalities like accessing metadata for music content and developing music visualizers.
These real-life examples illustrate the power of APIs and their wide-ranging applications across different sectors and platforms.
Troubleshooting Common API Issues
Regardless of their strength and adaptability, APIs may occasionally present obstacles. Some common issues include:
- Server-level issues
- Incorrect API requests
- Authentication errors
- Data format mismatches
- Rate limiting
- Versioning
- Caching errors
- Error handling
But fear not, there are effective ways to troubleshoot and debug API calls, starting with a single api call.
Tools like Moesif can be extremely helpful in this process. Used in conjunction with Postman, Moesif can provide valuable insights into API usage, helping developers identify and resolve issues more effectively.
Ultimately, addressing API issues often involves:
- Using HTTPS for secure communication
- Ensuring the correct usage of HTTP methods
- Gaining a deeper understanding of tips and techniques for troubleshooting API issues within your application.
Summary
We’ve journeyed through the world of APIs, exploring their basics, types, use cases, and potential challenges. APIs are the invisible enablers of our digital world, powering interactions, facilitating data sharing, and driving innovation. Whether you’re a developer, a business owner, or simply a curious learner, understanding APIs is a valuable skill in today’s interconnected world. As you embark on your own API journey, remember to select the right API for your needs, implement security measures, monitor your API usage, and never stop learning.
Start enhancing your API journey today by exploring Moesif’s extensive guides on building APIs. For a hands-on experience with Moesif’s analytics and monetization tools, sign up for a free trial or chat with our team of API experts to learn how Moesif can supercharge your API projects.