Python API Tutorial (Beginner’s Guide)
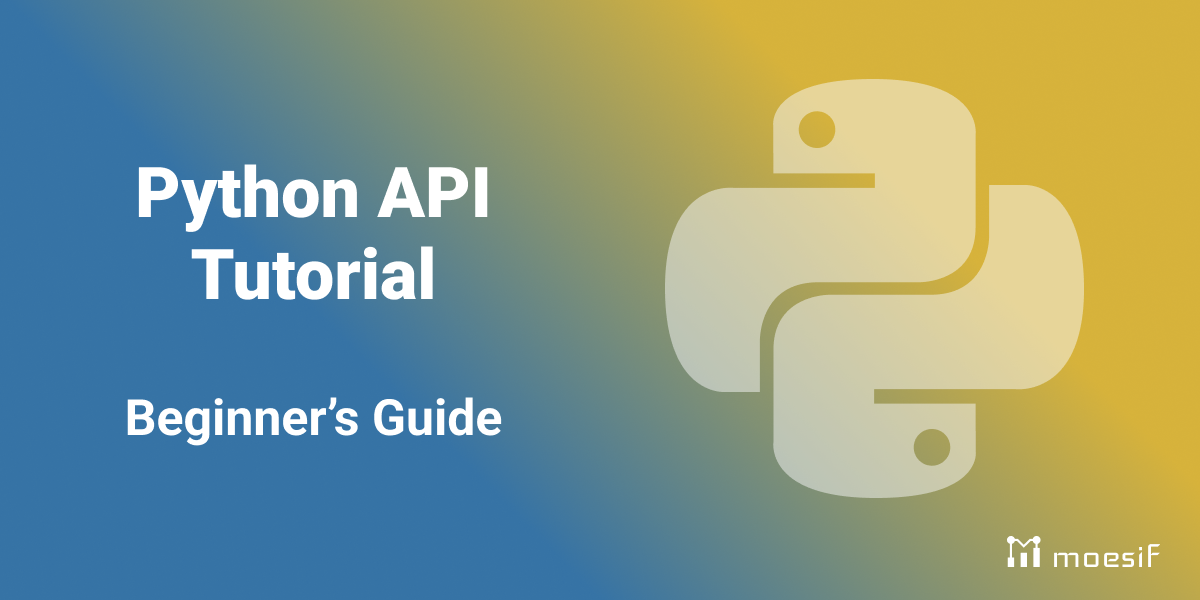
Introduction
When it comes to using and building APIs, Python applications are one of the most popular choices. Within the Python ecosystem, many different frameworks, such as Django and Flask, allow users to build out extensive API suites. Many people turn to staples such as Python Requests to use APIs within Python applications.
If you’ve landed on this blog, chances are you need to use APIs within your application and want to learn how to do so. Well, you’re in luck! In this blog, we will go over how to consume APIs within your Python application, status codes, and how to set query parameters, as well as how to work with JSON data that is getting sent to or received by an API call. Let’s begin with the basics by looking at exactly what an API is.
Introduction to APIs
As you are likely aware, API stands for Application Programming Interface. At its core, an API is a set of rules that allows different software applications to communicate with each other. It’s a bridge between different software systems, enabling them to interact and exchange data in a structured and secure manner. Users can send a request to the API, and the API will return some type of response.
To go a bit higher level, you can think of an API as a postal service for data. Similar to sending and receiving letters and packages through a postal service, software applications use APIs to send and receive data. The API defines the correct address format (the endpoint), the type of mail that’s allowed (the request type), and what you can expect to receive back (the response).
APIs power almost every facet of our digital lives in the real world. APIs have a wide range of uses that you are likely experiencing multiple, likely hundreds or thousands, times per day. Here are a few common examples:
-
Data Integration: APIs allow different databases or software services to share data. For instance, a weather application might use an API to gather weather data from a central database.
-
E-commerce: Online stores use APIs to connect with payment processors, ensuring smooth transactions.
-
Social Media Integration: Many websites and apps use social media APIs to allow users to sign in with their social media accounts, share content, or import their profile data.
-
IoT Devices: In the Internet of Things (IoT), smart thermostats and fitness trackers use APIs to communicate data to servers or other devices.
-
Cloud Computing: APIs are crucial in cloud services, allowing applications to access cloud resources and perform tasks like storing data or running computations.
APIs are critical in modern software development because they allow for the creation of flexible, modular software systems. By using APIs, developers can build upon the capabilities of existing services, speeding up the development process and enabling more complex functionalities. For example, a payment processing API, such as Stripe, could allow multiple companies to process payments without having to build such a service for themselves.
Getting Started with API Requests in Python
When using APIs within your Python code, you first need to figure out what type of API request you will be making. API requests can be of different types, commonly called methods. These methods each have a unique HTTP verb associated with them and a specific functionality based on that. Below are the four most common types.
- GET: To retrieve information.
- POST: To send new data.
- PUT: To update existing data.
- DELETE: To remove data.
You’ll want to select the correct endpoint and verb based on what type of action you are trying to complete through the API. For instance, we may have an endpoint called /users
that performs different functions depending on the type of request. If a GET
request is made, maybe a list of users will be returned, whereas if a POST
request is made, maybe a new user will be created based on the data received by the API.
Libraries for Making API Requests
For actually making API requests, Python has several libraries that can be used. Some of the most popular ones include:
- Requests: Known for its simplicity and ease of use. It’s an excellent choice for beginners and is widely used in the industry.
- HTTPx: An async-capable HTTP client similar to Requests but with async support.
- Urllib: A module available in Python’s standard library, useful for basic operations but less user-friendly compared to Requests.
When it comes to comparing these three libraries and deciding which one you may want to use, the choice is relatively easy. Essentially, all three of them will work for the basic operations we are talking about in this blog. However, as stuff becomes a little bit more complex, you might want to take a deeper look at the differentiators. Let’s look at those quickly below.
- Requests is often preferred for its simplicity and the readability of its code. It supports synchronous requests and is ideal for blocking I/O operations.
- HTTPx, on the other hand, is a more recent library that supports both synchronous and asynchronous requests, making it a good choice for high-performance applications.
- Urllib is part of the standard library but requires more code for everyday tasks and is less intuitive than Requests, making it less popular for new projects.
Adding Dependencies to Your Python Project
After you decide which library you want to use, you’ll need to include the dependency in your Python code. To use these libraries, you first need to add them to your Python project.
If you’re using Requests
, you can install it using pip, Python’s package manager. To do so, open your command line or terminal and run:
pip install requests
For HTTPx
, the installation command for pip
is:
pip install httpx
Since urllib
is part of the standard library, there’s no need to install it separately.
Then, in your code, you can import and use any of these libraries that you’ve added to your project. As an example, if you use Requests
, you can use it like so:
import requests
response = requests.get(‘https://api.com/test-service’)
Of course, the rest of this blog will focus specifically on using the Request
library. Next, let’s take a look at how to make a basic request to an API in more detail.
Initiating Our Initial API Request
Seeing the code in action when it comes to making a request to an API is one of the easiest ways to understand the concepts covered above. Let’s demonstrate a basic GET request using the Requests library. In the example below, the code queries a public API and then processes the API’s response.
import requests
# Replace with the desired API endpoint
url = 'https://api.example.com/data'
response = requests.get(url)
# Checking if the request was successful
if response.status_code == 200:
# Printing the retrieved data
print(response.json())
else:
print(f"Failed to retrieve data: {response.status_code}")
In this example, we send a GET request to an API endpoint, check if the request was successful by looking at the status code (which should be 200
if the request was successful), and then print the JSON response. Next, we will look at a slightly more advanced example where we can supply some data to the API through query parameters.
Integration of APIs with Query Parameters
When it comes to a developer’s skill-set, query parameters are a fundamental aspect of building API requests. Query parameters are appended to the URL of an API request and are used to modify the behavior of the request. Essentially, query parameters act as filters or additional instructions/data that the API can use to customize the response.
To add query parameters to an API request, you’ll need to use query parameter syntax, which requires a question mark (?
) followed by the query parameters you want to add. Multiple parameters are separated by an ampersand (&
). Below is an example of a URL with 2 query parameters.
https://api.example.com/data?parameter1=value1¶meter2=value2
Use Cases for Query Parameters
There are many scenarios where query parameters can be useful for API consumers and developers. Common uses of query parameters include:
- Filtering Data: Retrieve a subset of data based on given criteria.
- Sorting Data: Sort the returned data in a specified order.
- Pagination: Limit the number of results returned in a single request, usually for large datasets.
- Search: Query a dataset for specific terms.
- Configuring Responses: Customize responses, such as specifying a format.
Implementing Query Parameters in Python
Let’s consider a practical example where we use the Requests library in Python to make a GET request with query parameters. Suppose we are accessing an API that provides information about books, and we want to filter results based on the author and publish year. We could use the code below to filter out books by J.K. Rowling written in 1997.
import requests
url = 'https://api.example.com/books'
params = {
'author': 'J.K. Rowling',
'year': 1997
}
response = requests.get(url, params=params)
if response.status_code == 200:
books = response.json()
for book in books:
print(book)
else:
print(f"Error: {response.status_code}")
Line-by-line, the code above accomplishes the following:
- Imports the
requests
library. - Defines the base URL (
https://api.example.com/books
). - Create a dictionary called
params
with the required query parameters forauthor
andyear
. - Passes the URL and dictionary to the
requests.get()
function. - The Requests library then automatically constructs the correct URL with query parameters and sends the request.
- Processes the response based on the returned status and data.
This should give you a good idea of how query parameters can be used with Requests
in a very simple example. Of course, query parameters can be more or less complex depending on the use case.
Tips for Using Query Parameters
Now that we have covered the basics of using query parameters, we can chat about some tips and tricks to cover some nuances. Let’s review some of the most important ones below.
- Understanding the API: When using any API, especially those with query parameters, always refer to the API documentation. By referring to the docs, you’ll know which query parameters are supported, their format, and how they are expected to be used.
- Testing: When building requests with multiple query parameters, test them to ensure they work as expected and return the correct data. As more lengthy and complex query parameters are used in a request, there is a higher chance of issues with formatting and processing. Always ensure that requests work as expected, preferably with every possible query parameter combination (if there are multiple).
- Handling Special Characters: If you’re unaware, one of the most frustrating things is that certain characters may need to be encoded when used in query parameters. For instance, spaces are often encoded as
%20
or replaced with+
. Depending on the type of encoding the API is expecting, these values might be different. The easiest way to confirm is to look at an example in the API docs to see which characters should be encoded. - Default Values: Some APIs may have default values for specific query parameters. Understanding these defaults is crucial for interpreting the response correctly.
- Security: While query parameters are convenient for simple filters and configuration, sensitive information should never be sent through query parameters, especially in unencrypted HTTP requests. When creating your APIs or using third-party APIs, you should always be conscious of the data you send in a query param and if it is considered sensitive.
Real-World Example
Before moving on, let’s cover one last example that is more advanced. Consider an API where you must send a list of items as a query parameter. The API might expect the format to be comma-separated like this:
https://api.example.com/items?ids=123,456,789
In Python, you might construct this API request as follows:
item_ids = ['123', '456', '789']
params = {
'ids': ','.join(item_ids)
}
response = requests.get('https://api.example.com/items', params=params)
# Further processing of the response...
Here, ','.join(item_ids)
creates a single string from the list of item IDs, separated by commas, suitable for the query parameter. Many ways exist within Python and other languages to easily create requests with more sophisticated values than a single-value query string.
Decoding API Status Codes
As we have seen in some of the code above, when you make a request to an API, it responds with a status code. These status codes are part of the HTTP protocol and are standardized across the web, providing a quick way of understanding the result of your request. Essentially, they tell you if your request was successful or not. Specific status codes also denote an error; if an error occurs, the type of error will be reflected in the returned status code.
Status codes serve several purposes:
- Feedback: They inform the client (your program) about the result of its request.
- Troubleshooting: They help identify issues when a request doesn’t go as planned.
- Control Flow: In programming, developers can handle different scenarios based on the response status code.
The 5 Most Common HTTP Status Codes
Although many different HTTP status codes exist between 100 and 599, some are used much more frequently. Let’s look at a brief overview of five of the most common HTTP status codes you’ll likely see.
- 200 OK: This is the code you want to see. It means your request was successful, and the server is sending the requested data.
- 404 Not Found: The resource you tried to access doesn’t exist. This can happen if you mistype a URL or if the resource has been removed.
- 500 Internal Server Error: A generic error message indicating something has gone wrong on the website’s server. It’s not your fault but an issue on the server side.
- 401 Unauthorized: This status code appears when required authentication has failed or not been provided yet.
- 403 Forbidden: The server understands your request but refuses to authorize it. This could be due to a lack of permission to access the resource.
Handling Status Codes in Code
As we mentioned earlier, an advantage to having status codes is that they can be used to inform our applications about how a response should be handled. To demonstrate how this can be done in Python, below is a basic example using the Requests library to handle different status codes and print out a message accordingly.
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
print('Success!')
elif response.status_code == 404:
print('Resource not found.')
elif response.status_code == 500:
print('Server error.')
elif response.status_code == 401:
print('Unauthorized. Authentication required.')
elif response.status_code == 403:
print('Forbidden. Access denied.')
else:
print(f'Error: {response.status_code}')
Of course, the example above is extremely simple; it’s just printing some stuff to the console. However, this paves the way for more advanced logic where the application may retry the API call after 5 minutes if a 500
status code is returned or prompt the user for some credentials if a 401
error is brought back by a service.
Learning More About Status Codes
You can refer to detailed resources and posts for a deeper dive into the world of status codes and their meanings. At Moesif, we’ve created comprehensive guides and explanations that can help and other resources with great information on status codes. Here are links to articles from Moesif or other reliable sources to check out:
- Moesif’s Guide on HTTP Status Codes
- MDN Web Docs on HTTP Response Status Codes
- REST API Tutorial on HTTP Status Codes
These resources will provide more information on all the possible HTTP status codes, helping you understand and handle them more effectively as you come across them as you use APIs.
Navigating API Documentation
API documentation is essential for developers since it outlines how to use and integrate with an API effectively. Good documentation should include detailed information about the API’s endpoints, request methods, necessary parameters, and expected response formats.
There are typically two types of API documentation: docs generated through an OpenAPI spec and more typical API docs that are hand-written.
For OpenAPI-Generated documentation, these docs leverage OpenAPI (formerly Swagger), a specification for machine-readable API files. It allows for the generation of interactive documentation developers can use to understand and test an API directly from the browser. OpenAPI-generated docs often include an easy-to-navigate interface with expandable sections for each endpoint, showing required parameters, request examples, and response models.
More typical API documentation may vary in format but generally includes a comprehensive guide detailing aspects of the API, such as authentication, endpoints, parameters, and sample requests and responses.
Understanding API Documentation
Let’s consider a hypothetical example to understand how to navigate API documentation. Assume we are looking at documentation for a weather API. A section for the /weather
endpoint might look like this:
- Endpoint:
/weather
- Method: GET
- Query Parameters:
city
(required): Name of the cityunits
: Measurement units (metric
orimperial
)
- Response: JSON object containing weather details
Based on the above information, we can construct an API request. If we want to get weather data for London in metric units, our request will look like this in Python using the Requests library:
import requests
base_url = "https://api.exampleweather.com"
endpoint = "/weather"
parameters = {
'city': 'London',
'units': 'metric'
}
response = requests.get(base_url + endpoint, params=parameters)
if response.status_code == 200:
print(response.json())
else:
print(f"Error: {response.status_code}")
This request would then either bring back a response that contains the weather details for London, which would be printed out, or the call may return an error, in which case, we would log the error to the screen.
For a more advanced example, let’s consider a POST
request example where we must set a body field. Suppose we have an API for a task manager. The documentation for creating a new task is as follows:
- Endpoint:
/tasks
- Method: POST
- Body:
title
(required): Title of the taskdescription
: Detailed description of the task
- Response: JSON object with the details of the created task
Translating this into a request:
import requests
import json
base_url = "https://api.exampletaskmanager.com"
endpoint = "/tasks"
task_data = {
'title': 'Grocery Shopping',
'description': 'Buy milk, eggs, and bread'
}
response = requests.post(base_url + endpoint, data=json.dumps(task_data))
if response.status_code == 201:
print("Task created successfully:", response.json())
else:
print(f"Error: {response.status_code}")
In this POST request:
- We use
json.dumps
to convert thetask_data
dictionary into a JSON-formatted string. - The
requests.post
method sends the request, including the task data in the request body. - A status code of 201 typically indicates successful resource creation.
Tips for Navigating API Documentation
Although API documentation comes in all shapes and sizes, there are a few critical pieces to remember when using API docs to navigate onboarding a new API.
- Start with Authentication: Check how the API handles authentication (API keys, OAuth, etc.). If you do not use the correct authentication mechanism expected by the API, you’re guaranteed an unsuccessful API call and likely a
401
response code. - Understand Rate Limits: Look for any mention of rate limits to understand how many requests you can make in a given time-frame. Not all APIs have rate limits, but almost every public API will to prevent abuse and malicious behaviors such as a denial of service attack.
- Check Endpoints and Methods: Identify the available endpoints and what HTTP methods (GET, POST, etc.) they support. Some endpoints may support multiple methods, so use the proper method for your expected outcome.
- Review Request and Response Formats: Understand the format of requests you need to send and the responses you will receive.
- Look for Examples: Good documentation often includes sample requests and responses, which are very helpful. Most of the time, you can use these examples to build out your request without having to parse through many docs to find the same information.
Handling JSON Data in Python
If you work with RESTful APIs, you will work heavily with JSON. JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy to read and write for humans and easy to parse and generate for machines. JSON is popular because of its simplicity and flexibility. It’s language-independent, with parsers available for every programming language, including Python. Because of this, it has become a universal data format for APIs.
A JSON object is a collection of key-value pairs, where the key is a string, and the value can be a string, number, boolean, array, or even another JSON object. Below is an example of what JSON looks like.
{
"name": "John Doe",
"age": 30,
"isEmployed": true,
"skills": ["Python", "JavaScript", "SQL"]
}
Python has a built-in json
module that can be used for encoding and decoding JSON data. Using the json
library, let’s look at some examples of how you can work with JSON in Python
Parsing JSON
Known as deserialization, below is an example of how to convert a JSON string to a Python object.
import json
json_string = '{"name": "John Doe", "age": 30, "isEmployed": true}'
python_dict = json.loads(json_string)
print(python_dict)
Generating JSON
Known as serialization, below is an example of how to convert a Python object to a JSON string.
python_dict = {'name': 'Jane Doe', 'age': 25, 'isEmployed': False}
json_string = json.dumps(python_dict)
print(json_string)
Reading JSON from a File
Sometimes, you’ll want to read JSON from a file into your Python program. Below is an example of how to open a file and load JSON data using the json.load
function.
with open('data.json', 'r') as file:
data = json.load(file)
Writing JSON to a File
In the inverse, you may also want to write JSON data to a file. Below is an example of how you can do that by using the json.dump
function.
data = {'name': 'Jane Doe', 'age': 25, 'isEmployed': False}
with open('data.json', 'w') as file:
json.dump(data, file)
Working with JSON from an API
Often, you’ll receive JSON data as a response from an API. In this case, you can use the methods above to read and write JSON (to an API request or file). Below is an example of how JSON data from an API can be read using response.json
and then printed to the console.
import requests
import json
response = requests.get('https://api.example.com/data')
# Assuming the response contains JSON data
if response.status_code == 200:
data = response.json() # Converts JSON to a Python dictionary
print(data)
else:
print(f"Failed to retrieve data: {response.status_code}")
Python’s json
module, which we covered earlier, offers extensive support and functionality for more complex JSON operations, like parsing nested JSON data or handling exceptions. The examples above should cover many use cases you’ll encounter when working with APIs and JSON data in Python.
Python API Tutorial: Moving Forward
Now that we’ve covered all the bases on consuming APIs with Python, you may also be interested in building your own. For this, Moesif has created some extensive guides that show you how to build APIs with Flask and Django, two extremely popular Python frameworks developers use to build APIs.
In these guides, we walk through step-by-step how to build your own APIs and show you how you can leverage Moesif to track API usage, errors, and dig into user behavior. Check them out to get started on creating your own APIs with Python.
Conclusion
We’ve covered a lot of ground in this blog, starting from the basics of what an API is, diving into making API requests with Python, handling JSON data, understanding query parameters, and decoding status codes. By now, you should have a solid grasp of how to consume APIs within your Python application, an essential skill in today’s interconnected digital world.
While consuming APIs is crucial, creating and managing your own APIs can take your projects to the next level. Whether you’re looking to build APIs for internal use or as products for your customers, understanding the lifecycle of an API - from creation to management - is key.
To aid in this journey, Moesif offers a suite of tools that can enhance your experience with APIs. With Moesif, you can gain insights into how your APIs are used, monitor for errors, and understand user behavior. This is invaluable for making data-driven decisions to improve your APIs. If you’re considering turning your APIs into a product, Moesif provides features to help you monetize them effectively. By tracking API usage, you can integrate with billing providers and set up a pricing model that aligns with your business strategy.
Start enhancing your API journey today by exploring Moesif’s extensive guides on building APIs with popular Python frameworks like Flask and Django. For a hands-on experience with Moesif’s analytics and monetization tools, sign up for a free trial or chat with our team of API experts to learn how Moesif can supercharge your API projects.