Build Great APIs with These Essential REST API Best Practices
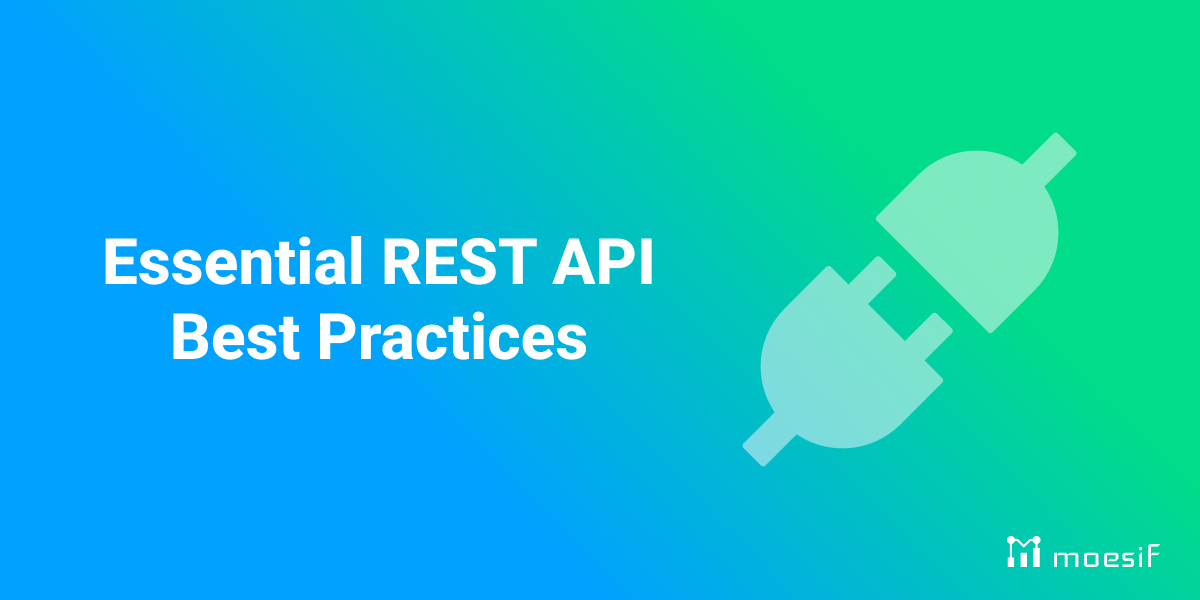
When it comes to REST API best practices, it can be hard to understand whats important and what is not. This means that developers need immediate, applicable guidance when it comes to applying best practices for building great APIs. This article will dive into techniques that optimize REST API design for clarity, stability, and speed. Discover how strategic endpoint crafting, judicious HTTP method usage, and meticulous attention to security can revolutionize your API. This guide is your concise roadmap to building web services that developers love and that you can efficiently maintain.
For the tl;dr, here are a few of the key takeaways from what we will cover in the blog:
- REST API design requires precise endpoint naming using noun-based, pluralized resource names and restricted levels of nesting for clear and easy navigation, along with proper use of HTTP methods for CRUD operations and appropriate status codes for communication and error handling.
- Security measures, including robust authentication and authorization strategies, data encryption, and SSL/TLS implementation, are crucial to protecting a REST API against threats and ensuring data integrity.
- To optimize performance and usability, REST APIs should incorporate effective caching, rate limiting, detailed documentation with versioning best practices and real-world use-cases, along with client-side resources such as SDKs, libraries, and testing environments.
Now, let’s get started by looking at how to dial in your RESTful API design and bring your APIs to the next level.
Designing REST API Endpoint Names
In the realm of REST APIs, the design of your endpoints can make or break your API’s success. Scalability, reliability, and flexibility in REST API design start with ensuring clear and concise endpoint naming. Imagine being a developer and having to navigate through a maze of confusing and inconsistent endpoint names. Frustrating, right? Hence, the building block of enhancing your API’s design and developer experience begins with the use of precise naming conventions and the correct HTTP methods for what an endpoint does. Let’s dig into a few best practices to apply on this front.
Nouns Over Verbs
When designing your API endpoints, using nouns in your URIs instead of verbs can make a world of difference. Why? Because nouns specify the resources and align with the concept of resources and collections, providing a clear structure for developers to interact with your API. On the other hand, verbs can lead to a long and inconsistent list of endpoints, making your API design complex and counter-intuitive.
Example:
- Verb-based endpoint: /api/getBooks or /api/createBook
- Noun-based endpoint: Instead, use /api/books for retrieving books and POST to /api/books to create a new book. This approach makes it clear that the action is on the “books” resource, aligning with REST principles.
Pluralize Resource Names
While we’re on the topic of resource names, it’s worth discussing the importance of pluralizing resource names. Pluralizing REST API resource names clarifies that the endpoint can return a collection of entities. This simple practice can save your API users from unnecessary confusion.
Example:
- Singular resource name: /api/book might imply it deals with a single book, which can be misleading.
- Pluralized resource name: /api/books clearly indicates that the endpoint is designed to return multiple books or manage books as a collection, enhancing clarity.
Nesting Resources for Relationships
Another important aspect of API design is nesting resources to reflect the relationships between entities. In REST APIs, nesting should reflect the natural hierarchy and relationships between entities, aiding users in understanding the relations between different endpoints. However, it’s important to limit this nesting to one level to improve clarity and avoid complexity.
Example:
- Without nesting: Having separate endpoints like /api/books and /api/authors might not clearly show the relationship between books and their authors.
- With nesting: /api/authors/123/books indicates that you’re accessing the books related to the author with ID 123. This nested structure clearly shows the relationship between authors and their books, making it easier for API users to understand how to access related resources. However, remember to keep nesting to one level where possible to maintain simplicity and avoid overly complex endpoint paths.
Leveraging HTTP Methods and Status Codes
Leveraging HTTP methods and status codes is just as important as precisely naming endpoints. HTTP methods are utilized in RESTful APIs to perform actions on resources, each method representing specific CRUD operations. Additionally, HTTP response codes give valuable information to clients, indicating the status of their request, whether it was successful, or received a client or server-side error.
By utilizing these tools correctly, API developers can enhance an API’s consistency and ease of use.
CRUD Operations with HTTP Methods
The primary HTTP methods used for CRUD operations in REST APIs are:
- POST: used to create new resources
- GET: used to retrieve a representation of a resource
- PUT: used to update or create a resource
- DELETE: used to delete a resource
These methods ensure that your API’s functionality is specified in the HTTP request method. Looking back at our points on naming, using the correct method negates the need for verbs in the endpoint name.
Proper Usage of HTTP Status Codes
HTTP status codes are more than just numbers; they are crucial in communicating the results of a REST API call. To improve error handling, APIs should use appropriate HTTP status codes to inform clients about the success or failure of their requests. For instance, a 200 - Success
status code indicates that the request has succeeded, whereas a 400 - Bad Request
status code indicates a client-based error.
Effective communication with users about the outcome of their requests improves the overall experience developers have when using an API. This highlights the benefits that can be achieved through the use of appropriate status codes.
Error Handling with Status Codes
Beyond being communicative tools, status codes are also vital for error handling in REST APIs. Providing detailed error responses using appropriate status codes is an effective way to communicate with users about the outcome of their requests. For instance, status codes in the 400-499 range can inform the client about issues such as invalid requests, authentication failures, and permission issues, while a 500 status code indicates a generic server error.
Robust error handling ensures your API remains user-friendly and easy to navigate, even in case of errors. Many frameworks and languages that developers use to consume APIs also work more effectively when status codes are used for error handling.
Implementing Query Parameters and Pagination
Query parameters and pagination are two features that can significantly enhance the control and usability of web APIs, especially REST APIs. Query parameters enable actions such as filtering, sorting, and pagination on the collection of data retrieved by a REST API. Let’s dig into the particulars a bit further.
Path Parameters vs. Query Parameters
Understanding the difference between path and query parameters can greatly enhance your REST API design. While path parameters form part of the URL’s path, query parameters are optional and are used for creating more complex or informative requests. For example, path parameters are used for operations such as deleting or updating a specific resource, whereas query parameters are better suited for requests that sort or filter the request data.
Path Parameters:
- Use Case: Identifying a specific resource.
- Example: To retrieve a specific user’s profile, the URL might look like /api/users/123, where 123 is the path parameter indicating the user ID.
Query Parameters:
- Use Case: Filtering, sorting, or further refining requests.
- Example: To search for users by name and sort them by their registration date, the URL might be /api/users?name=John&sortBy=registrationDate&order=asc. Here, name, sortBy, and order are query parameters used to filter and sort the data.
Filtering and Sorting with Query Parameters
Query parameters come in handy when you want to filter and sort the requested data in your REST API. They enable the filtering of data by criteria such as date ranges or categories, improving data retrieval for users. Additionally, sorting data is facilitated through a query parameter by specifying the order and field to sort by, such as ascending or descending.
Filtering Example:
- Suppose you have an API endpoint for fetching orders. To retrieve orders placed within a specific date range, the request might look like /api/orders?startDate=2022-01-01&endDate=2022-01-31. Here, startDate and endDate are query parameters used to filter orders by their placement date.
Sorting Example:
- To sort these orders by price in descending order, the URL might be /api/orders?sortBy=price&order=desc. In this case, sortBy specifies the field to sort by (price), and order specifies the direction of sorting (descending).
The implementation of these practices enhances user control over retrieved data, thereby making your API more flexible and user-friendly.
Pagination Techniques
When dealing with large datasets, implementing effective pagination techniques is crucial. With pagination, the client can dictate the size of data pages and the starting point of the dataset they wish to receive, enhancing control and usability. Various techniques, including page-based, time-based, and cursor-based pagination, can effectively handle large datasets and ensure consistency.
Page-based Pagination Example:
- For page-based pagination, if you want to retrieve the third page of a list of books, with ten books per page, the request might look like /api/books?page=3&limit=10. Here, page indicates the page number, and limit specifies the number of items per page.
Time-based Pagination Example:
- To fetch news articles published after a certain timestamp, the request could be /api/articles?publishedAfter=2022-07-01T00:00:00Z. Though not a traditional pagination technique, it’s akin to cursor-based pagination where publishedAfter acts as a cursor for time.
Cursor-based Pagination Example:
- For cursor-based pagination, if you’re retrieving the next set of user activities after a specific activity ID, the URL might be /api/activities?cursor=abc123&limit=20. Here, cursor is the unique identifier (ID) of the last activity in the previous fetch, and limit controls how many subsequent activities to return.
Implementing the right pagination technique guarantees the efficiency and user-friendliness of your API, even when handling large amounts of data.
Enhancing API Security
In a world where data breaches are increasingly common, enhancing the security of your REST API is absolutely necessary. To protect against API threats, it’s essential to have robust authorization alongside encryption and authentication measures. This includes practices such as implementing SSL (secure socket layer) to ensure secure data transfers and reduce vulnerability to attacks.
Authentication Mechanisms
Authentication mechanisms are vital in verifying the identity of clients interacting with your API. This includes methods like usernames/passwords, OAuth tokens, and json web tokens (JWT). Implementing these mechanisms effectively can enhance the security of your API and ensure only authorized users have access to your API’s resources.
Authorization Strategies
Beyond authentication, implementing effective authorization strategies is crucial in managing user permissions and access rights in REST APIs. This includes practices like role-based access control (RBAC) and attribute-based access control (ABAC), which simplify the management of access rights by assigning permissions to roles. Implementing these strategies helps to ensure that your API and data remains secure and only accessible to the right users.
Encryption and Secure Data Transfer
Data encryption is another crucial aspect of API security. Using TLS and HTTPS, you can encrypt data in transit and ensure secure communication between clients and servers. To go further, sensitive data should be encrypted not only in transit but also at rest. Implementing encryption in both scenarios helps to safeguard against unauthorized access and prevents data breaches.
Optimizing API Performance
Ensuring optimal performance of your REST API is essential for a seamless user experience. This can be achieved through various techniques such as caching, rate limiting, and throttling. By applying these strategies, you can improve the performance and responsiveness of your API, even under high loads.
Caching Strategies
Caching is a powerful technique that can greatly improve your API’s performance. This involves storing cached data in the server’s RAM or using server-side caching techniques like database queries and stored calculations for rapid data retrieval on subsequent requests. Effective caching strategies contribute to reducing server load and maintaining the speed and efficiency of your API.
Rate Limiting and Throttling
Rate limiting and throttling are essential measures to prevent overuse and ensure your API remains responsive and scalable under high loads. These techniques involve limiting the number of requests a client can make in a certain period, thereby protecting your API from resource exhaustion and targeted DDoS attacks. As much a piece of API performance as it is API security, enforcing rate limiting and throttling guarantees a fair usage policy and maintains a high quality of service for all users.
Monitoring and Analyzing API Performance
Monitoring and analyzing your API’s performance is vital for maintaining its quality and reliability. This involves keeping track of key metrics like:
- Uptime
- CPU and memory usage
- API consumption
- Response time
- Error rate
- Unique API consumers
By regularly monitoring these metrics, you can identify and address any performance issues early on, ensuring your API remains efficient and reliable.
Creating Comprehensive API Documentation
Many believe that an API is only as good as its documentation. Since documentation can be a big reflection on the overall API experience, comprehensive API documentation is vital for increasing the adoption and ease of use for APIs. This includes providing clear and detailed instructions for using your API, as well as maintaining up-to-date information about changes and updates.
Importance of API Documentation
API documentation is more than just a manual for using an API—it’s a tool that can significantly improve the developer experience and increase user adoption. Comprehensive API documentation contributes to the awareness of the API via the network effect, with satisfied users advocating for the API. Clear and user-friendly documentation reduces the time and costs associated with support, making your API more appealing to developers. Having documentation which accurately shows how users can build a request and what to expect in a response is extremely important for developers.
Versioning Best Practices
Versioning is also a crucial aspect of API management. It allows you to manage changes to your API and maintain compatibility across different versions. This can be done in several ways:
- Incorporating the version number into the URI path
- Using query parameters to determine the API version
- Sending a specific version number in the API request headers.
Adopting versioning best practices ensures the reliability and consistency of your API, even with the introduction of new features or changes to existing ones.
Client-Side Considerations
While the server-side plays a crucial role in REST API design, it’s important not to overlook client-side implementations. These should focus on real-world use cases and provide environments such as sandboxes for testing.
Considering client-side requirements and providing appropriate resources ensures that your API is robust on the server-side and user-friendly on the client-side.
SDKs and Libraries
SDKs (Software Development Kits) and Libraries are comprehensive toolkits that assist developers in various stages of the application development process, including handling HTTP/HTTPS requests. They offer a level of abstraction that hides the complexities of underlying technologies, enabling easier integration into various products. By providing comprehensive SDK documentation with sample code, you guide developers through different implementation scenarios and reduce their learning curve versus using the API directly.
Use Case-Oriented Documentation
Use case-oriented documentation is another important aspect of client-side considerations. This type of documentation should emphasize the benefits and opportunities provided by the API, offering a narrative that showcases how it can enhance products or simplify developers’ workflows. Clear and detailed use case-oriented documentation ensures that your API is robust, user-friendly, and easy to understand.
Sample Code and Testing Environments
Providing sample code and testing environments can greatly enhance the developer experience. Sample code guides developers through different implementation scenarios and reduces their learning curve. Likewise, testing environments like API sandboxes allow for testing and development to occur simultaneously, thereby accelerating development cycles. Providing these resources guarantees that your API is not only robust and efficient, but also easy to implement since end-to-end examples can be easily understood and used as a base for implementation.
Avoiding Common API Design Mistakes
Even with the best practices in hand, mistakes can happen. However, being aware of the most common API design mistakes can help you prevent them from occurring in your own API design. These mistakes include inconsistent naming conventions, poor error handling, and ignoring security best practices. Being aware of these pitfalls and working to avoid them ensures your API remains robust, secure, and reliable.
Inconsistent Naming Conventions
Inconsistent naming conventions can lead to a confusing and frustrating experience for developers using your API. It’s important to use consistent, clear, and intuitive naming conventions across your REST API endpoints to maintain predictability and ease of integration. By avoiding special characters, unsafe ASCII characters, file extensions, and inappropriate abbreviations in URIs, you can ensure your API remains simple to use and navigate.
Poor Error Handling
Poor error handling can lead to confusion and frustration for your API users. This can occur when your API:
- Responds with multiple errors in a non-standardized format
- Overlooks the inclusion of a detailed explanation or user-friendly message
- Returns inconsistent API responses
Robust error handling and clear, detailed error messages ensure your API remains user-friendly and easy to navigate, even in case of errors.
Ignoring Security Best Practices
Ignoring security best practices in your API design can lead to serious consequences, including data breaches and unauthorized access to your API’s resources. This could occur if you fail to conduct a comprehensive risk assessment for your APIs or overlook the recording of your APIs in a dedicated registry. Adhering to security best practices and maintaining up-to-date records of your APIs, including proper management of api keys, ensures that your API remains secure and reliable.
Summary
As we’ve seen, designing a successful REST API involves a lot more than just writing code. From designing precise endpoints and leveraging HTTP methods to implementing effective caching strategies and providing comprehensive documentation, there are countless best practices to consider. By adhering to these principles and avoiding common pitfalls, you can ensure that your REST API is not only robust and efficient, but also secure, user-friendly, and easy to implement.
When it comes to monitoring and maintaining your APIs, Moesif’s API analytics and monetization solution is a great asset. Moesif can help to give insights into how users are using your APIs, allow you to identify issues in onboarding and spikes in errors, and help you monetize your APIs in a matter of minutes. To try it out for yourself, sign up and integrate in minutes to begin monitoring and exploring your API data.