How to Choose the Best Javascript Data Visualization Library
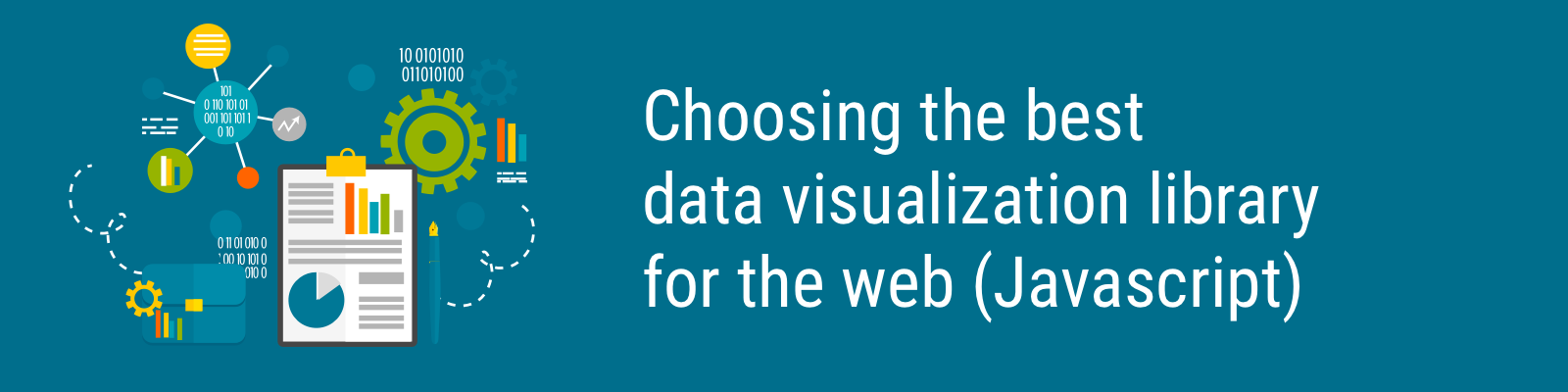
Any data focused application needs data visualization for the UI/dashboard. For web-based applications, those libraries are almost always Javascript. Getting the charts and visualizations right can be hard, but luckily, we have a variety of Javascript libraries to choose from such as D3.js, Chart.js, and Google Charts. However, there can be an overwhelming number of choices with pros and cons for each library. This guide can help you when choosing a library along with some best practices.
Factors to Consider
Browser & Device Compatibility
This probably is one of the most important factors. While it is worth considering which browser and versions are supported by a library, but if you try to support really old version of browsers it may make you not able to take advantage of the latest features. You can look at the browser market share to decide where to draw the line.
If your app will be used beyond web and on Mobile web, check to see if the charts can be responsive and make sure it looks good on mobile browser.
UI Framework Compatibility
Modern web application front ends are usually developed using one of the major UI frameworks, such as Ember, React, Vue, or Angular.
Depends on the framework you are using, you may want to ensure that the library plays well with it. Most of the charting libraries are framework agnostic. And often, there are wrapper for each major framework. However, there are quirks with each framework that may worth verifying, as some libraries assumes that the code will only be executed in the client-side environment. As more and more modern frameworks now support server side rendering, it may require special hacks to make sure that the client side only libraries are not loaded on the server side.
Wide Range of Chart Types
There are some charting libraries that only focus on one or two types of charts, such as Timeline series or directional graphs, but most libraries trying to be comprehensive in the set of graphs it has. The best comparison is on the Wikipedia.
If one library doesn’t have all the chart types your need, it doesn’t hurt to use two or three charting libraries, but you do probably want to make them visually style consistent if they will be part of the same application.
File Size
The Javascript library needs to be downloaded to the clientside as part of your application. To have a good user experience, you want to reduce the size of your Javascript as much as possible.
So the combined size of the Library is important. There are several ways to mitigate this.
- You can try to do code splitting wisely or delay the loading of each libraries until it is needed. Webpack and other tools often easy ways of handling this.
- If you only have needs for one or two types of charts, it may not make sense to load a large library that supports many charts.
- Some libraries offers the option to load only the chart types you want.
- If you are using
import
, sometimes you can load specific components that you need instead of the entire library.
Performance
If you plan to render large data sets at one time, then performance will matter. You can try to benchmark, but the libraries that are designed for large data sets usually will call out the performance as their main features.
Style and Customization
For aesthetics, it is a more subjective judgement call regarding how pretty it is. In this article, I’ve shared some of my personal opinions.
Some libraries support animations while others do not, and quality of animation vary. Although if you chose to use animation, be sure to add in moderation, as it can be very distracting.
The most important aspect regarding visuals is probably customization, i.e. how much you have control over the style so that you can fit the theme and styles of your application.
Price, License and Support
Some of the most comprehensive charting libraries also require a paid license, often they offer free trials or free for non-commercial uses. For the few I looked at, the prices seem comparable.
For free libraries, they vary from free to use vs completely open source under MIT or Apache license. They all are provided with no warranties.
One of the benefits for paid products is that they will offer support, but for free libraries, you will most likely depend on the community. If the community is big enough (you can take a look at the number of stars received on Github), usually there is a lot of people who are willing to help. A big plus of big community is that the library is less likely to get abandoned.
Popular Free Libraries
Wikipedia provides a very good feature comparison table of all the Javascript charting libraries. Here, I’ll just go into some of the most popular ones.
Chartjs
This is one of most popular. It is under MIT license, which is one of the least restrictive licenses.
The range of chart types isn’t huge, only 8, but they are very easy to work with.
Visually, it looks very pretty with a minimalistic and flat look, right out of the box. The customization is somewhat limited compared to others. The default animation is very well done. It is also responsive.
It has a very large community, so there are a lot of wrappers for major frameworks like React.
With a small footprint, it is a great for getting started. But it is only designed
for client-side use, so if you are using server-side rendering, you have to be sure to
not require
it on the server side.
Google Charts
Among free visualization libraries, Google Charts offers one of largest sets of chart types. Besides the typical line, bar, and pie charts, it offers many less commonly used ones like Candlestick Chart, Calendar Chart, Geochart, Org Charts and Tree Map Charts.
Even though Google Charts is free, but it is not open source, it follows Google API terms of use. With Google as its backer, it is unlikely to become abandoned.
Visually, it doesn’t have that clean and minimalistic look right out of box compared to Chartjs. But you can customize it. Number of customization parameters varies by chart type, but there is a lot of different things to play with. Although it isn’t responsive right out of the box, you can write code to resize the graph that detects the event when the window is resized or container is resized.
Unlike other libraries, it does not support npm
or bower
installation, which
is disappointing.
Rather, it requires including a loader script via script
tags from their CDN. Unlike some
other libraries, you use the loader to dynamically load the package with the chart types you need, and
then render your graph within the finished loading callback: onLoadCallback
.
This can make the library sometimes a little awkward to use, especially if you have other triggers that you want to use for rendering. Luckily, there are also wrappers for react.
D3.js
D3.js is the golden standard for data visualization in Javascript, it often is the foundation for other libraries. It is open source and free, under the BSD license.
It has a reputation for steep learning curve, and no charts are provided from the base library. But there is a vast community of users who support each other and answer questions, and I personally find this library pretty easy and fun to use.
There are some libraries build on top such as react-d3, and C3js that provides about the same set of chart types out of the box as chartjs, i.e. the basic ones like: Line, Bar, Area, Scatter, Pie.
If you have a special need for visualization, such as new or unique type of chart, D3 is probably the right solution. Still, unless you have to, do not reinvent the wheel, first check if there is another library that meets your needs first.
Paid Commercial Libraries
These libraries often offer free trial or free license for personal use, but require a fee for commercial use. If you require support beyond hoping for answers from the community, they might be good choices. The market leaders seem to be Highchart, Fusionchart, amCharts and Anychart. They all offer large range of chart types with a lot of customization, integration options, and popular frameworks support. Go ahead and check them out.
Visualization Libraries for Specific Frameworks
Most libraries do not rely on other UI frameworks. Most are designed in to
render to a div
tag. Since there are many frameworks, such as JQuery, Ember,
Angular, or React, some people create wrappers for these libraries (e.g. react-chartjs and react google charts mentioned earlier). However, some wrappers are awkwardness to
use, and some are not kept up to date, and you end up add one more
layer of dependency.
However, there are some charting libraries specifically designed for the popular front end frameworks, they might be easier to work with if you are already using the framework and they have chart types that you need.
- Flot is very specific to jQuery.
- Ember Charts is built on top of D3.js for emberjs framework.
- n3 charts, again built on top of D3.js, but built for the Angular framework.
- Victory is designed specifically for react.
- React-Vis is designed specifically for react also, it comes from the Uber team.
Specific One Chart Type Focused Libraries.
There are free libraries that focus on one type of chart that does a super good job that I would like to call out.
- Sigmajs creates beautiful graph drawings, I.e. representation of nodes and networks.
- Springy.js specializes in force directional graphs that offers a very “springy” animation.
- Smoothie Charts focuses on displaying streaming data.
Conclusion
The number of choices in Javascript visualization libraries can be overwhelming, but that is a reflection of how important data visualization is for web applications. We are excited to see that all the major libraries are constantly improving.
This article is not exhaustive, so let me know in the comments below if you have additional thoughts on factors that should be considered when choosing a library and what libraries you really liked.