Django REST API Tutorial: The Ultimate Guide
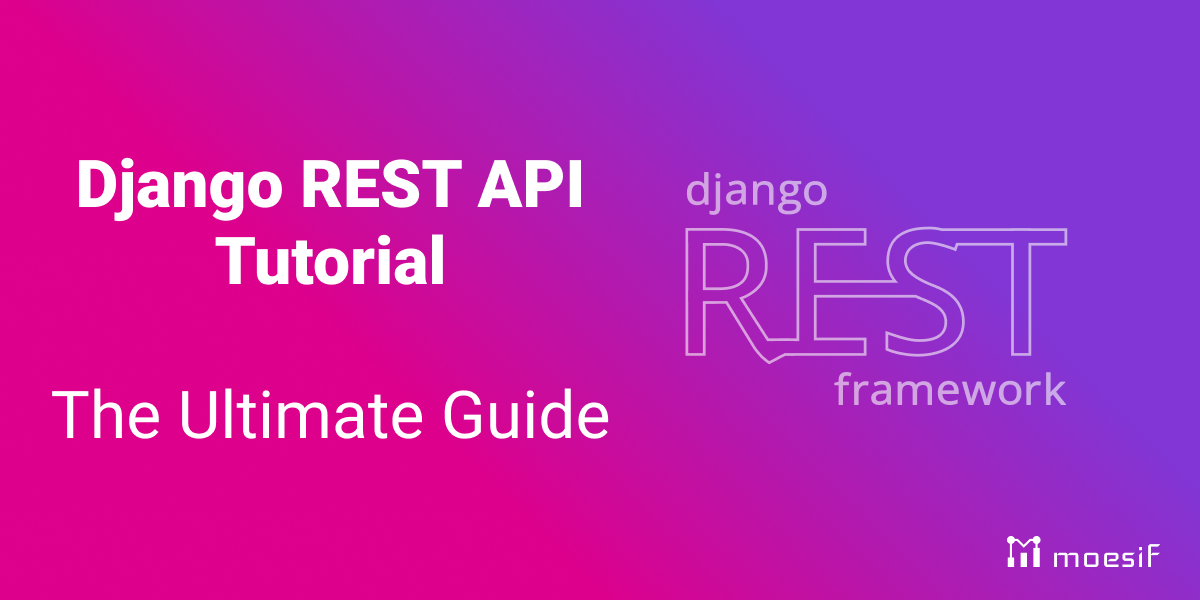
Are you ready to start exploring the world of API development with Django? In this tutorial, we will begin an exploration of how to leverage Django together with the Django REST framework, a robust and versatile framework for creating efficient and scalable APIs. This guide is designed to provide you with a clear and structured approach to Django REST API, ensuring that both beginners and experienced developers can navigate and utilize this powerful tool with ease. Join us as we delve into the intricacies of building a Django REST API with the REST API framework, transforming your understanding and skills in API development.
What is Django?
Django is an open-source web framework. It is written in Python and known for its simplicity, flexibility, and robust features. It follows the “batteries-included” philosophy, providing an all-encompassing set of tools and features required for web development right out of the box. This includes components for handling database operations, URL routing, HTML templating, and security features, among others. Designed to facilitate rapid development and clean, pragmatic design, Django helps developers avoid many common pitfalls of web development, thereby streamlining the process of building scalable and maintainable web applications. Its emphasis on reusability and “pluggability” of components, less code, low coupling, and the principle of Don’t Repeat Yourself (DRY) makes it a popular choice for both beginners and experienced developers in building a wide range of web applications, from small-scale projects to large-scale enterprise solutions.
What is a REST API?
A REST API, known formally as a Representational State Transfer Application Programming Interface, is a software architectural style for creating and offering web services. It facilitates communication between various software applications over the internet through standard HTTP methods like GET, POST, PUT, and DELETE. In a RESTful architecture, both data and functionality are treated as resources, accessible via Uniform Resource Identifiers (URIs), commonly in the form of web links. These resources are manipulated using stateless operations, meaning the server does not need to remember any previous interactions and each request from a client contains all the information needed to process it. REST APIs are crafted to be lightweight, straightforward, and scalable, which contributes to their popularity in web services and as a foundation for the backend development of web and mobile applications. They often return data in formats like JSON or XML, allowing for easy integration with various types of clients.
What makes Django REST framework a compelling choice?
Django REST framework (DRF) stands out as a compelling choice for building web APIs due to its powerful and flexible toolkit that seamlessly integrates with the Django web framework. It offers a clean, simple, and rapid development approach, making it highly efficient for creating RESTful APIs. One of the key strengths of DRF is its browsable API feature, which greatly enhances developer productivity and debugging processes by allowing API endpoints to be easily explored and tested directly from the web browser. Additionally, DRF provides a comprehensive set of features including serialization for ORM and non-ORM data sources, extensive support for class-based views, and a wide range of authentication and permission policies. Its modularity and customizability enable developers to tailor the framework to fit specific project requirements, while its adherence to the DRY (Don’t Repeat Yourself) principle helps in reducing code redundancy. The framework’s robust documentation and active community support further add to its appeal, making it a top choice for developers looking to build high-quality, maintainable, and scalable RESTful web services.
Setting Up Django REST Framework
Step 1: Setting Up Your Python Environment
Before building your API, it’s important to set up your development environment correctly. This step ensures that all the necessary tools and packages are in place, paving the way for a smooth development process. Here’s how to get started:
-
Install Python: Django is a Python-based framework. That means we need to make sure that Python is installed on your system. Python 3 is recommended as it offers better support and more features. You can download Python from the official Python website. During installation, make sure to select the option that adds Python to your PATH variable, which makes it accessible from the command line.
-
Set Up a Virtual Environment (Optional but Recommended): Virtual environments are always a good idea, and that goes for Django projects as well. This isolates your project and its dependencies from other projects, preventing any conflicts. To create a virtual environment, navigate to the directory where you want your project to be and run:
python -m venv myenv
To activate the virtual environment, use:
- On Windows:
myenv\Scripts\activate
- On macOS and Linux:
source myenv/bin/activate
Your command line will show the name of the virtual environment, indicating that it’s active.
- On Windows:
-
Installing Django: Once Python is ready, your next move is to install Django. This is effortlessly achieved using Python’s package manager, pip. Execute the command below:
pip install django
This will download and set up the most recent version of Django.
-
Adding Django REST Framework: To build Web APIs with Django, the Django REST Framework is indispensable. It provides an extensive selection of tools to reduce the friction of creating a Django REST API from scratch. Install it using pip:
pip install djangorestframework
-
Verify the Installation: After installing Django and Django REST Framework, it’s good to verify that everything is set up correctly. You can do this by running:
python -m django --version
This command should return the version of Django that’s installed on your system. Similarly, you can check the Django REST Framework version in your Python shell:
import rest_framework
print(rest_framework.__version__)
With these steps, your environment is now ready for developing APIs using Django REST API. This setup provides a solid foundation for the rest of the tutorial, where you’ll start building your actual API.
Step 2: Creating a Django Project
Once your environment is set up, the next step is to create a new Django project. This project will act as the home base for your API. Here’s how to get started:
-
Create a New Django Project: A Django project encapsulates the configurations and settings for a group of Django applications. To create a new project, run the following command in your terminal or command prompt (make sure your virtual environment is activated if you’re using one):
django-admin startproject myproject
Replace
myproject
with your desired project name. This command creates a new directory with your project name and sets up a basic Django project structure within it. -
Understanding the Project Structure: After running the command, you’ll notice a new directory with the name of your project. Inside this directory, you’ll find several files:
manage.py
: This is a command-line tool that facilitates various interactions with your Django project. -myproject/
: Named after your project, this subdirectory houses your project’s actual Python package. -myproject/__init__.py
: A blank file that signals Python to treat this directory as a Python package. -myproject/settings.py
: Contains all the settings and configurations for your Django project. -myproject/urls.py
: This file is tasked with defining the URL patterns of your project.myproject/asgi.py
andmyproject/wsgi.py
: These files are used for deploying your project to a web server.
-
Modify Settings: Open the
settings.py
file in your project directory. Here, you can adjust various settings like time zone, static files path, installed apps, middleware, etc. For now, you’ll need to add ‘rest_framework’ to theINSTALLED_APPS
section to include Django REST Framework in your project:INSTALLED_APPS = [ ... 'rest_framework', ]
-
Run the Development Server: To check if everything is set up correctly, you can run Django’s development server. Go to the command line, navigate to your project directory (where
manage.py
is located), and run:python manage.py runserver
This command starts a development server on your local machine. You can visit
http://127.0.0.1:8000/
in your web browser to see the default Django welcome page. This confirms that your project has been set up successfully. -
Initial Migration: Before starting development, it’s a good practice to run the initial database migrations. Django uses these migrations to set up a database schema. Run the following command:
python manage.py migrate
This command applies the default migrations that come with Django, setting up your database with the necessary tables.
With these steps, you have successfully created a new Django project and are ready to proceed to the next phase of building your API with the Django REST API framework. This foundation is crucial as it sets the stage for the development of your API endpoints and data models.
Step 3: Create a Django App
After setting up your Django project, the next step is to create a Django app. An app in Django is a web application that does something – e.g., a blog, a database of public records, or a simple poll app. A project can contain multiple apps, and an app can be in multiple projects. Here’s how to create your first app within your Django project:
-
Creating the App: To create a new app, you need to run a command in the terminal (ensure you are in the directory containing
manage.py
). Use the following command:python manage.py startapp myapp
Replace
myapp
with your desired app name. This command creates a new directory inside your project with the name of your app, containing several Python files and a subdirectory. -
Understanding the App Structure: The newly created app directory will have the following structure:
migrations/
: This directory stores database specific information related to your models.__init__.py
: An empty file that tells Python that this directory should be considered a Python package.admin.py
: Here, you can register your models to include them in the Django admin site.apps.py
: This file is used for app-specific configurations.models.py
: This file is used to define your app’s data models.tests.py
: You can write test cases for your app here.views.py
: This file is used to handle the request/response logic for your web application.
-
Register the App: To include your app in the project, you need to register it in the
settings.py
file of your project. Open thesettings.py
file and add your app to theINSTALLED_APPS
list:INSTALLED_APPS = [ ... 'myapp', ]
-
Plan Your App: Before diving into coding, it’s a good practice to plan out what your app will do. For instance, if you’re creating an API, you should define what resources it will expose, what data models it will use, and how the API endpoints should behave.
-
Create Models: If your app will be using a database, this is the time to define your models in
models.py
. Models in Django are Python classes that define the structure of your database tables and the behavior of the data stored within them. -
Initial Migration for Your App: Once you have defined your models, you should create and apply migrations for them. Run the following commands:
python manage.py makemigrations myapp python manage.py migrate
These commands create new migration files (based on the models you defined) and apply these migrations to the database, creating the necessary tables.
By following these steps, you have successfully created a Django app within your project. This app will serve as a component of your project where you can develop specific functionalities, such as the API endpoints you’ll be creating using the Django REST API framework.
Now, with your app set up and registered, you can start developing its functionalities. This typically involves writing views, defining URLs, and creating templates (if your app has a frontend component).
Setting up Django Rest framework
Step 4: Model Your Data
Modeling your data is a crucial step in building an API with Django REST API. This involves defining the structure of the data your application will handle. In Django, models are Python classes that define the fields and behaviors of the data you’re storing. Essentially, each model maps to a single database table.
Here’s how to model your data effectively:
-
Understanding Django Models: In Django, a model is the single, definitive source of information about your data. It contains the essential fields and behaviors of the data you’re storing. Django follows the DRY (Don’t Repeat Yourself) principle, so the goal is to define your data model in one place and then derive things from it.
-
Define Your Models: In your app directory, open the
models.py
file. This is where you will define your models. For example, if you are creating a blog API, you might have a model for blog posts:from django.db import models class BlogPost(models.Model): title = models.CharField(max_length=200) content = models.TextField() published_date = models.DateTimeField(auto_now_add=True) def __str__(self): return self.title
In this example,
BlogPost
is a model with three fields -title
,content
, andpublished_date
. Django provides a variety of field types to represent different types of data. -
Field Types and Options: Django models offer a range of field types and options. Some common field types include:
CharField
for character fieldsTextField
for large text fieldsDateTimeField
for dates and timesIntegerField
,DecimalField
, andFloatField
for numbers
Each field type in Django comes with various options you can use to customize its behavior, such as
max_length
forCharField
,auto_now_add
forDateTimeField
to automatically set the field to the current date and time when the object is created, etc. -
Model Methods: You can also define methods within your model. In the example above, the
__str__
method is used to return a human-readable representation of the model, which is helpful in Django’s admin interface and shell. -
Making Migrations: After defining your models, you need to create migrations for them. Migrations are Django’s way of propagating changes you make to your models (adding a field, deleting a model, etc.) into the database schema. Run the following commands:
python manage.py makemigrations myapp python manage.py migrate
The
makemigrations
command tells Django that you’ve made some changes to your models and that you’d like to store these changes as a migration.migrate
applies the migrations to the database. -
Admin Interface: To add your model to the Django admin interface, you need to register the model in the
admin.py
file of your app:from django.contrib import admin from .models import BlogPost admin.site.register(BlogPost)
This step is optional but highly recommended, as it provides a convenient, GUI-based way to interact with the data.
By following these steps, you have successfully modeled your data in Django. This model will act as the blueprint for your database, allowing Django to create the necessary database tables and providing a structured way to interact with the data through your API.
Step 5: Migrate Your Models
After defining your models in Django, the next crucial step is to migrate these models to create the corresponding database schema. Migrations in Django are a way of applying changes you make to your models (like adding a field, deleting a model, etc.) into the database structure. Here’s how to effectively handle migrations:
-
Understanding Migrations: Migrations are Django’s way of propagating changes in your models (like adding a new field, deleting a model, etc.) into the database schema. They are designed to be mostly automatic, but you’ll need to know when to make migrations and when to run them.
-
Making Migrations: Once you have defined or updated your models, you need to tell Django to create migrations for these changes. Run the following command:
python manage.py makemigrations myapp
Replace
myapp
with the name of your app. This command creates new migration files - which are Python scripts - in themigrations
folder of your app. These files are named automatically with a timestamp to help you identify the order of migrations. -
Review Migration Scripts: Before applying the migrations, it’s a good practice to review the migration scripts that Django has generated. This can be particularly important in a production environment or when working on complex data models. You can find these scripts in the
migrations
folder of your app. -
Apply Migrations: To apply the migrations to your database, run:
python manage.py migrate
This command looks at all available migrations and applies those that haven’t been applied yet to your database, synchronizing the changes you made in your models with the schema in the database.
-
Migration Dependencies: Sometimes, your migrations depend on each other, especially when you’re working with multiple apps. Django takes care of these dependencies, but it’s important to be aware of them, especially when rolling back migrations or when you have complex database schemas.
-
Rolling Back Migrations: If you need to undo a migration, you can use the
migrate
command with the name of the app and the migration you want to revert to. For example:python manage.py migrate myapp 0001
This command will revert all migrations up to (and including) 0001_initial.py
for myapp
.
-
Using Migrations in a Team Environment: When working in a team, it’s important to regularly pull and push migrations. Conflicts in migrations can occur and need to be resolved carefully, usually by discussing with the team and deciding on the correct order of migrations.
-
Migrations and Version Control: Migrations should be included in your version control system. They represent important changes and history of your database schema and are necessary for other team members and for deployment.
By following these steps, you ensure that your database schema is always in sync with your Django models. Migrations are a powerful feature of Django that facilitate the evolution of your database schema over time without requiring you to drop your database and lose data.
Step 6: Create a Serializer
After setting up your models and applying migrations, the next step in building your API with Django REST API is to create serializers. Serializers in Django REST Framework are responsible for converting complex data types, such as querysets and model instances, to native Python datatypes that can then be easily rendered into JSON, XML, or other content types. They also provide deserialization, allowing parsed data to be converted back into complex types, after first validating the incoming data.
Here’s how to create and work with serializers effectively:
-
Understanding Serializers: Think of serializers in Django REST Framework as similar to Django Forms. Like forms, serializers handle both the conversion of data to and from Python datatypes and validation of incoming data. They are an essential component in a Django REST API for data handling.
-
Define Your Serializer: In your Django app, create a new file named
serializers.py
. This is where you will define your serializers. For each model, you typically create a corresponding serializer. For example, if you have aBlogPost
model, you can create aBlogPostSerializer
:from rest_framework import serializers from .models import BlogPost class BlogPostSerializer(serializers.ModelSerializer): class Meta: model = BlogPost fields = ['id', 'title', 'content', 'published_date']
In this example,
BlogPostSerializer
is aModelSerializer
that automatically generates a set of fields for you, based on the model. TheMeta
class inside the serializer class specifies which model it should serialize and which fields should be included. -
Field Types in Serializers: Just like models, serializers can define various field types. The Django REST Framework provides a set of field classes that map closely to Django’s model fields (like
CharField
,IntegerField
, etc.). You can also define custom fields for more complex data handling. -
Validation in Serializers: Serializers also handle validation. You can define custom validation methods for your serializer fields or override the
.validate()
method to add any specific validation logic for your serializer. -
Nested Serializers: Sometimes, you might need to include related objects in your serialized output. Django REST Framework allows you to nest serializers within each other. For example, if your
BlogPost
model has a foreign key to aUser
model, you can create aUserSerializer
and include it in yourBlogPostSerializer
. -
Read-Only and Write-Only Fields: In some cases, you might want certain fields to be read-only or write-only. This can be specified in your serializer fields using the
read_only=True
orwrite_only=True
arguments. -
Using Serializers in Views: Once you have defined your serializers, you will use them in your views to handle the incoming and outgoing data. Serializers will convert querysets and model instances to JSON data that can be returned in HTTP responses, and they will also handle parsing and validating JSON data sent by clients.
By following these steps, you create a bridge between your complex data types (like model instances) and the JSON data that is sent and received in your API. Serializers are a powerful feature of Django REST Framework that streamline the process of data serialization and deserialization, making it easier to build robust and efficient APIs.
Creating API Views in Django
Step 7: Set Up Views
In Django REST Framework, views are where you define the logic of your API. They determine how to process incoming requests and return responses. For a RESTful API, you typically need to handle various HTTP methods like GET, POST, PUT, and DELETE. In Django, these methods are referred to as actions. The actions include list, create, retrieve, update, and destroy. Here’s how to set up your views effectively, including examples for each of these methods:
-
Understanding ViewSets: Django REST Framework introduces the concept of ViewSets, which are classes that provide the logic for handling requests. They are a high-level abstraction over the traditional Django views and are particularly suited for standard database operations. They reduce the amount of code you need to write to create API endpoints.
-
Creating a ViewSet: In your
views.py
file, you can create a ViewSet for your model. For example, if you have aBlogPost
model and a correspondingBlogPostSerializer
, your ViewSet might look like this:from rest_framework import viewsets from .models import BlogPost from .serializers import BlogPostSerializer class BlogPostViewSet(viewsets.ModelViewSet): queryset = BlogPost.objects.all() serializer_class = BlogPostSerializer
This
BlogPostViewSet
class will automatically providelist
,create
,retrieve
,update
, anddestroy
actions. -
Handling GET Requests (List and Retrieve): The
list
action in a ViewSet handles GET requests to the root of the URL, returning a list of all instances. Theretrieve
action handles GET requests to a specific instance, like/api/blogposts/1/
, returning that particular instance. -
Handling POST Requests (Create): The
create
action in a ViewSet handles POST requests. It allows clients to create a new instance of a model. The data sent in the request body is validated against the serializer and, if valid, a new instance is created and saved to the database. -
Handling PUT Requests (Update): The
update
action handles PUT requests. It is used to update an existing model instance. The request URL specifies the instance to update, and the request body contains the updated data. -
Handling DELETE Requests (Destroy): The
destroy
action handles DELETE requests. It allows clients to delete an existing instance. The request URL specifies which instance to delete. -
Customizing ViewSet Behavior: While ViewSets provide a lot of functionality out of the box, you might need to customize their behavior. You can override action methods like
create()
,update()
, ordestroy()
to add custom logic. -
Example of a Custom Action: Suppose you want to add a custom action to your
BlogPostViewSet
that allows users to like a blog post. You can do this using the@action
decorator:from rest_framework.decorators import action from rest_framework.response import Response class BlogPostViewSet(viewsets.ModelViewSet): # ... existing code ... @action(detail=True, methods=['post']) def like(self, request, pk=None): blogpost = self.get_object() # Add logic to like the blog post return Response({'status': 'blog post liked'})
This custom action
like
will be available at a URL path like/api/blogposts/1/like/
.
By setting up your views in this manner, you create a robust and flexible API that can handle various types of requests. Django REST Framework’s ViewSets and the ability to customize them provide a powerful way to build efficient and maintainable APIs.
Step 8: Configure URL Routing
After setting up your views, the next step in building your Django REST API is to configure URL routing. This involves mapping URLs to your views so that the correct view is called for each endpoint. Django REST Framework offers a straightforward way to handle URL routing, making it easy to map resources to their corresponding views.
Here’s how to configure URL routing effectively:
-
Understanding Django URL Dispatcher: In Django, the URL dispatcher is used to direct HTTP requests to the appropriate view based on the request URL. It uses a URLconf, which is a set of patterns that Django tries to match the requested URL to find the right view.
-
Setting Up URL Patterns: In your Django project’s
urls.py
file (located in the project’s main directory), you will include the URL patterns for your app. First, you need to import the necessary functions and include your app’s URLs. For example:from django.urls import include, path from rest_framework.routers import DefaultRouter from myapp.views import BlogPostViewSet router = DefaultRouter() router.register(r'blogposts', BlogPostViewSet) urlpatterns = [ path('', include(router.urls)), ]
In this example, a
DefaultRouter
is used for automatic URL routing to your views. Therouter.register
method connects the URL pattern to the viewset. -
URL Patterns for ViewSets: When you use a router, it automatically generates the URL patterns for the standard actions in your viewsets (like
list
,create
,retrieve
,update
, anddestroy
). For example, theBlogPostViewSet
will have URL patterns for listing all blog posts, retrieving a single blog post, creating a new blog post, etc. -
Custom URL Patterns: If you have custom actions in your viewsets (like the
like
action in the previous example), the router will also generate appropriate URL patterns for these actions. -
Namespacing URL Patterns: If your project contains multiple apps, it’s a good practice to namespace your URL patterns. This means including the app’s URLs under a specific namespace:
urlpatterns = [ path('api/', include((router.urls, 'myapp'), namespace='myapp')), ]
This allows you to reverse URLs unambiguously in templates and view functions.
-
Testing Your URLs: After setting up your URL patterns, you should test them to ensure they correctly map to your views. Django’s shell and test framework can be useful for testing URL configurations.
-
URL Design Considerations: When designing your URLs, consider RESTful principles. For instance, use simple, readable URLs that reflect the nature of the data being accessed or manipulated. Also, use nouns instead of verbs in your URL paths (e.g.,
/blogposts/
instead of/get_blogposts/
).
By configuring your URL routing properly, you ensure that your Django REST API is well-structured and that each endpoint is correctly mapped to its corresponding view. This step is crucial for the functionality of your API, as it defines how clients interact with your application and access its resources.
Step 9: Implement Authentication and Permissions
In building a robust Django REST API, implementing authentication and permissions is a critical step. This ensures that only authenticated users can access certain endpoints, and users can only perform actions they’re permitted to. Django REST Framework provides a flexible authentication and permissions system that can be tailored to your needs.
Here’s how to implement authentication and permissions effectively:
-
Understanding Authentication and Permissions: Authentication determines the identity of a user, while permissions determine if an authenticated user has access to perform a certain action. Django REST Framework supports various authentication schemes like Basic Authentication, Token Authentication, and OAuth.
-
Setting Up Authentication: To set up authentication, you need to choose an authentication scheme and configure it in your Django settings. For example, to set up Token Authentication, you first need to add
'rest_framework.authtoken'
to yourINSTALLED_APPS
and runpython manage.py migrate
to create the necessary database tables. Then, in yoursettings.py
, add:REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': [ 'rest_framework.authentication.TokenAuthentication', ], }
-
Implementing Permissions: Permissions are used to grant or deny access to different parts of your API. In your views, you can set permission classes to control access. Django REST Framework provides a set of built-in permission classes like
IsAuthenticated
,IsAdminUser
, andIsAuthenticatedOrReadOnly
. For example:from rest_framework.permissions import IsAuthenticated from rest_framework import viewsets class BlogPostViewSet(viewsets.ModelViewSet): permission_classes = [IsAuthenticated] # rest of the viewset code
This will ensure that only authenticated users can access the endpoints defined in
BlogPostViewSet
. -
Custom Permissions: If the built-in permissions don’t fit your needs, you can define custom permission classes. A custom permission class is a Python class that extends
rest_framework.permissions.BasePermission
and overrides the.has_permission()
and/or.has_object_permission()
methods. -
Token Generation and Handling: For token-based authentication, when a user logs in, a token is generated and sent back to the user. This token must be included in the
Authorization
header of HTTP requests to access protected endpoints. -
Securing Sensitive Information: Be cautious with sensitive information. Ensure that tokens and credentials are transmitted securely, preferably over HTTPS. Also, be mindful of storing sensitive data on the client side.
-
Testing Authentication and Permissions: It’s important to test your API’s authentication and permissions to ensure that they are working as expected. You can write tests to check if the API responds correctly to authenticated and unauthenticated requests, and if the permissions are correctly enforced.
-
Third-Party Packages for Extended Functionality: If you need more advanced authentication features, like OAuth or social authentication, you can use third-party packages like
django-rest-auth
ordjango-allauth
.
By implementing authentication and permissions, you add a layer of security to your Django REST API, ensuring that only authorized users can access and modify data. This is a crucial aspect of any API, especially when dealing with sensitive or personal data.
Step 10: Thoroughly Test Your API
Testing your Django REST API is an essential step to ensure its functionality, reliability, and security. It involves a series of checks and validations to make sure that your API behaves as expected under various conditions. Here’s an expanded guide on how to thoroughly test your API:
-
Start Your Development Server: Before testing, you need to run your development server. Use the command
python manage.py runserver
in your terminal. This will start the server, typically accessible athttp://localhost:8000/
. -
Manual Testing with Browser and Tools: Initially, you can perform manual testing by visiting endpoints in your browser. For example, navigate to
http://localhost:8000/blogposts/
to test your blog posts API. For more complex requests (like POST, PUT, DELETE), or to test headers and authentication, use tools like Postman or cURL. These tools allow you to craft specific HTTP requests and inspect the responses. -
Automated Testing: Automated testing is crucial for maintaining long-term quality and reliability. Django’s test framework allows you to write test cases in Python. Create a
tests.py
file in your app directory and write test classes that inherit fromdjango.test.TestCase
. Test different aspects of your API, including:- Testing HTTP Methods: Write tests for GET, POST, PUT, and DELETE requests. Ensure that your API responds with the correct status codes and data.
- Authentication and Permissions: If your API uses authentication, write tests to verify that unauthenticated requests are properly rejected and that users have the correct permissions for different actions.
- Edge Cases and Error Handling: Test how your API handles invalid inputs, unexpected request formats, and other edge cases. Your API should respond with appropriate error messages and status codes.
-
Writing a Sample Test Case: Here’s an example of a test case for creating a blog post:
from django.urls import reverse from rest_framework import status from rest_framework.test import APITestCase from .models import BlogPost class BlogPostTests(APITestCase): def test_create_blogpost(self): """ Ensure we can create a new blog post. """ url = reverse('blogpost-list') data = {'title': 'Test Post', 'content': 'Test content'} response = self.client.post(url, data, format='json') self.assertEqual(response.status_code, status.HTTP_201_CREATED) self.assertEqual(BlogPost.objects.count(), 1) self.assertEqual(BlogPost.objects.get().title, 'Test Post')
-
Continuous Integration (CI): Implementing CI practices, such as using GitHub Actions or Jenkins, can help automate the testing process. These tools can run your test suite on every push or pull request, ensuring that changes do not break existing functionality.
-
Performance Testing: If your API is expected to handle high traffic, conduct performance testing. Tools like Apache JMeter or Locust can simulate multiple users accessing your API to identify performance bottlenecks.
-
Security Testing: Ensure your API is secure against common vulnerabilities. This includes testing for SQL injection, data leaks, and proper authentication and authorization checks.
-
Documentation and Test Cases: Keep your test cases well-documented. This documentation serves as a valuable resource for understanding the expected behavior of your API and can be particularly helpful for new developers joining the project.
By following these steps and regularly running tests, you can catch bugs early, prevent regressions, and maintain the overall health of your Django REST API. Remember, a well-tested API is a cornerstone of a reliable and trustworthy application.
Conclusion
Django REST API framework is a powerful tool for your web development projects, whether small or large. To elevate your API’s performance and gain valuable insights, integrate Moesif with your Django projects. Moesif offers real-time analytics, event logging, and user behavior analysis, essential for optimizing your API and enhancing user experiences.
For a seamless integration process, follow our Django integration guide. And don’t stop there – sign up for Moesif today and unlock the full potential of your Django APIs.