Implementing API Analytics with Java
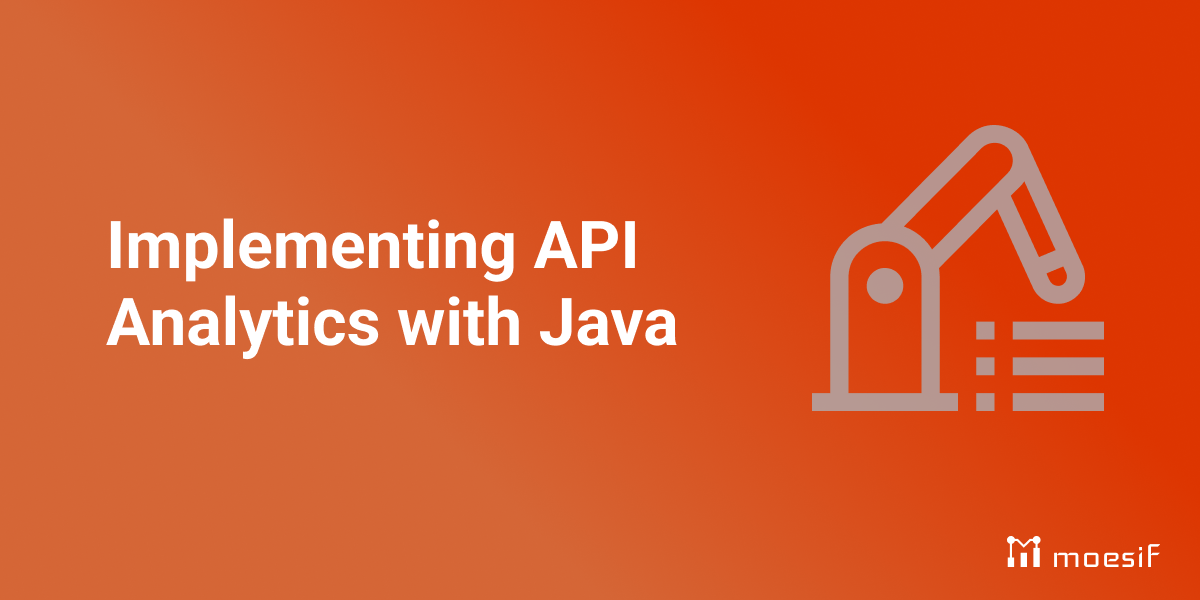
There are few technologies as ubiquitous – and crucial for business success – as APIs. APIs connect different software systems together, forming a common language that allows for substantial portability, scalability, and extensibility.
What is just as important as the systems themselves is understanding the systems and discovering insights about their usage. API analytics play a vital role in delivering this insight, providing context around the performance, usage patterns, and overall health of these APIs. Java, with its robust ecosystem, offers various tools and frameworks that can be leveraged to implement effective API analytics. This article aims to explore these tools and methodologies in detail.
Understanding API Analytics
Before delving into the specifics of Java, it’s crucial to understand what API analytics actually entails. API Analytics has two core processes – setting the metric and tracking the metric. Effective tracking of these metrics includes collecting the data, analyzing the data, and reporting the data, and each stage of this process requires some thought into the form and function of the systems involved.
Setting the Metric
When choosing what metrics to track, it’s important to figure out the relevancy of the data to your business logic and use case. While it would be great to have total insight into every possible metric at all times, this can introduce too much information, resulting in the conversion of data and information into pure noise. This noise can result in a reduction of value in the analytics and context generated by this process.
Accordingly, there are a few categories to consider when deciding on the metrics you track.
- Volumetric Data – this data involves information about the number of requests made, the total data transferred, etc. This information can help you understand how the API is used and to what extent, and can help identify high-traffic systems and endpoints.
- Service Data – this information can include data around how long it takes for an API to respond, how efficient these responses are, and so forth. This is especially useful to track over time to see what the incidence of failure or inefficiency across the ecosystem of offerings.
- User Data – this data includes user behavior and sentiment, and can be tracked through ongoing API data collection and then enriched with consumer support systems.
- Error Data – this data is relevant to the errors generated by the API, and can help set a standard for the health of the overall systems.
Each of these categories include metrics that could be argued into other categories, but understanding them in this format is a great start. There are as many metrics you can track as there are systems to track them!
Tracking the Metric
Once you have established what metrics you wish to track, you must actually start to track them. There are a variety of amazing tools in the Java ecosystem that can deliver powerful API analytics. Let’s take a look at some of the most popular ones.
Micrometer
Spring Boot Actuator is a part of the Spring Boot framework. It provides built-in endpoints for monitoring and interacting with your application, and can be easily integrated into your Java application to provide valuable insights into the runtime behavior of your application. Actuator connects to a collection facade called Micrometer, which provides real-time monitoring and analytics. Micrometer directly connects to the following monitoring systems:
* Netflix Atlas
* CloudWatch
* Datadog
* Ganglia
* Graphite
* InfluxDB
* JMX
* New Relic
* Prometheus
* SignalFx
* StatsD
* Wavefront
Getting Started with Micrometer
Getting started with Micrometer is quite easy – a more in-depth guide is located on the Springboot website, but is adapted here for brevity.
In order to use Micrometer, you’re going to need to create an application pair with a service application and a client application. The service application will allow you to utilize a properties file to set some variables – notably, you’ll be able to use it to set a port and to set an integration for your analytics engine of choice. In the tutorial from Springboot, they target Wavefront, an analytics dashboard for data insights. This properties file looks roughly like this:
spring.application.name=service server.port=8083 wavefront.application.name=console-availability management.metrics.export.wavefront.source=my-cloud-server
From here, you will create your client application. This application will allow the service application to be exercised, calling the data in question and pushing it to Wavefront.
Some key details of the Spring Boot approach should be considered. Firstly, Micrometer, though billed as an analytics system, is more a facade over the instrumentation part of Spring Boot – for this reason, you can use Micrometer to push to any solution (including something like Moesif). This gives you a ton of flexibility in finding the right combinatory stack of offerings. While you certainly can utilize Micrometer as a very basic analytics engine in and of itself, you’re going to need a database solution like Prometheus or Datadog to store this data, and it’s advisable to use a visualization solution to get the most benefit out of this stored data.
Moesif
Moesif is a world-class insights and analytics platform that can help you deploy an effective metrics system at scale with very little overhead. Moesif operates in real-time, providing analytics powered by context and observation rather than just network information or base data.
This can unlock an incredible amount of value for most users in and of itself, but additional support for monetization and revenue generation solutions can deliver significant monetary benefits as well. For Java implementations specifically, Moesif supports many Java server solutions, making implementation quite quick and easy!
Getting Started with Moesif
Connecting a Java development to Moesif is quite easy. By using Spring Boot, we can utilize a very simple deployment system.
First, you need to install the SDK. This is quite easily done, with support via Maven:
<dependency>
<groupId>com.moesif.servlet</groupId>
<artifactId>moesif-servlet</artifactId>
<version>1.7.5</version>
</dependency>
And support via Gradle:
dependencies {
compile 'com.moesif.servlet:moesif-servlet:1.7.4'
}
With these dependencies in place, all you have to do is install the Moesif Filter object, using your Moesif Application Id to connect to the service:
import com.moesif.servlet.MoesifFilter;
import javax.servlet.Filter;
import org.springframework.web.servlet.config.annotation.*;
import org.springframework.context.annotation.*;
import org.springframework.http.converter.*;
@Configuration
public class MyConfig extends WebMvcConfigurerAdapter {
@Bean
public Filter moesifFilter() {
return new MoesifFilter("Sign in to get your Moesif Application Id");
}
}
That’s it! You are now connected to Moesif in Java!
ELK Stack (Elasticsearch, Logstash, and Kibana)
The ELK Stack is another very popular solution for API analytics. Named after its components – Elasticsearch, Logstash, and Kibana – the ELK Stack is a combinatory solution that leverages each component for a specific function:
- Elasticsearch – A search and analytics engine commonly used for log data storage, search, and analysis.
- Logstash – Used for log collection, enrichment, and transportation.
- Kibana – Provides visualization capabilities for data stored in Elasticsearch, making it easier to create dashboards and visual reports.
Getting Started with the ELK Stack
Getting started with the ELK Stack is going to vary depending on your specific language, your tech stack, and your operating environment. Generally speaking, the process will require you to install each component individually, and then configure them to speak with each other by using the service as a common endpoint. There are many services which bundle the ELK Stack together as a single offering – for instance, logz.io provides this service, allowing you to get started pretty quickly compared to manually building. The drawback to this approach is you are buying into a product and ecosystem, and jumping out of this can be difficult.
Implementing API Analytics in Java
Regardless of your chosen solution, there’s a general process to implementing Java that should be kept in mind as you deploy at scale. This process generally looks as follows.
Step 1: Setting Up a Java Project for Analytics
To begin building API analytics, you must first set up your Java project to use a good analytics solution. Many solutions, such as Micrometer, require a set of dependencies to integrate properly. During this stage, reviewing the dependencies to ensure proper configuration is vital. Ensure that you have a long-term view of what this support looks like. Solutions like Micrometer are quite low-effort, but the trade off is generally for power and ease. The ELK Stack is much more powerful, but also requires a lot of overhead and time with more complex environments.
Step 2: Configuring the Analytics Tools
Ensure you have properly configured your tools. This involves reviewing your properties files, yes, but it also requires a deep introspection as to how you handle data. This is critical to ensuring security – misconfiguration drives many data exposures, so properly configuring is good business and good ethics. Do your due diligence!
Step 3: Collecting Metrics
At this stage, you’ll need to consider how you’re collecting metrics. What database solution you use, and how this data is stored and encrypted at rest, is going to determine how complex the rest of this process is, so consider this a critical point of failure. Make sure your metrics collection is efficient to prevent impact on production service from the API.
Step 4: Analyzing the Data
Choose your monitoring solution to visualize and analyze data. This is going to be a decision largely driven by business needs, so make sure that your business logic is sound and that your needs are clearly-defined – analyzing data can be expensive, but it’s even more so when you’re analyzing things your business doesn’t even care about!
Step 5: Make Data-Driven Decisions
Now that you have your metrics, use it to make data-driven decisions! This requires surfacing as much as you can as often as you can, but it also involves making this data readily accessible. Make sure you are not siloing your data.
Challenges and Best Practices
Challenges
The biggest challenge you are likely to run into with Java API analytics is in data overhead. For a long time, Java had a reputation of being a slow language, and while this is not really true in 2023, it still utilizes quite a bit of memory in delivering speed. Accordingly, API analytics can introduce a memory drain that could degrade API performance if not properly implemented.
Avoiding this issue is going to require creating your solution with an eye for efficiency in design. Metrics should only be collected once, and should be transformed separately from the collection stage. They can also play double duty – some metrics can represent multiple things, allowing you to get insights by collecting only a single data point.
Additionally, there are some privacy and security concerns that come with any metric approach. Depending on what data is being sent, it’s possible that PII or other data could be logged as part of a Java API analytics deployment. Ensure that you are sanitizing data, or, if such data cannot be sanitized, ensure that you are logging to a secure resource that is only accessible by trusted teams.
Best Practices
Efficiency is a big part of this equation. Efficiency will determine the value of the context you generate – after all, analytics is worth very little when it costs you excessively in terms of resources to get it.
Developers should consider scalability. Logging and analytics are easy to do when you’re handling ten or fifteen requests a day, but when you are handling hundreds of thousands of requests a minute, this can change dramatically. Ensure that your analytics system is built for efficiency and that you are logging the correct amount of information. If your approach is not verbose enough, you run the risk of doing analytics work that does not actually provide context or information. If it is too verbose, you run the very serious risk of running into resource crunch that occurs exponentially with little warning.
Most importantly, ensure that you are utilizing a trusted system for logging and analytics. Moesif is a powerful solution that is built on efficiency and security, and as such, stands above the crowd as a feature-complete toolset that can easily be integrated into your Java stack. If you are using another solution, you must audit the solution to ensure that it complies with your security posture, that it does not introduce inefficiency, and that the libraries and frameworks it depends on are secure and efficient in their own right.
Why Moesif?
There are quite a few third party solutions that you can use, but Moesif stands above the rest. Moesif delivers the highest potential for success with the lowest amount of friction, and can see adoptees get started within a matter of minutes.
It should be noted that the use of a third party solution begs the question – why not do it in-house? Doing in-house analytics, while possible for most developers, introduces a lot of long-term management in addition to immediate upfront cost. This also requires reinventing a solution that has already been effectively developed elsewhere – you’re doubling the cost for very little potential.
Adopting a trusted third-party solution can short circuit this issue. Moesif is a highly powerful solution that can provide an incredible amount of benefit with very little downside. In addition to the powerful suite of analytics on offer, Moesif also provides systems for monetization, observability, and effective billing, which unlocks huge potential revenue sources for APIs of any size.
Moesif is very easy to get started with – there are many options for integration for a wide array of languages and frameworks, and most new users could get started in minutes.
Moesif | Other Solutions |
---|---|
Easy to deploy with wide integration support | Often limited to specific languages or frameworks |
World-class analytics and metrics for high visibility and business metrics | Typically limited to surface-level network traffic |
Powerful monetization with deep billing provider integration | Decoupled monetization and billing – often one or the other |
Flexible pricing without surprises | Pricing can be highly variable |
Extensions gallery offers new features with low overhead | Extensibility often requires more complexity and third party solutions |
Complete all-in-one package for business success | Only handles one or two parts of your business success plan |
Conclusion
The implementation of API analytics is one of the best returns on investment the average developer can deploy. Using some common sense strategies and some trusted systems, developers can unlock logging and monitoring at scale, delivering effective analytics that can play a crucial role in the scalability and extensibility of their service.