Step-by-Step Blueprint to Create RESTful APIs for Efficient Web Services
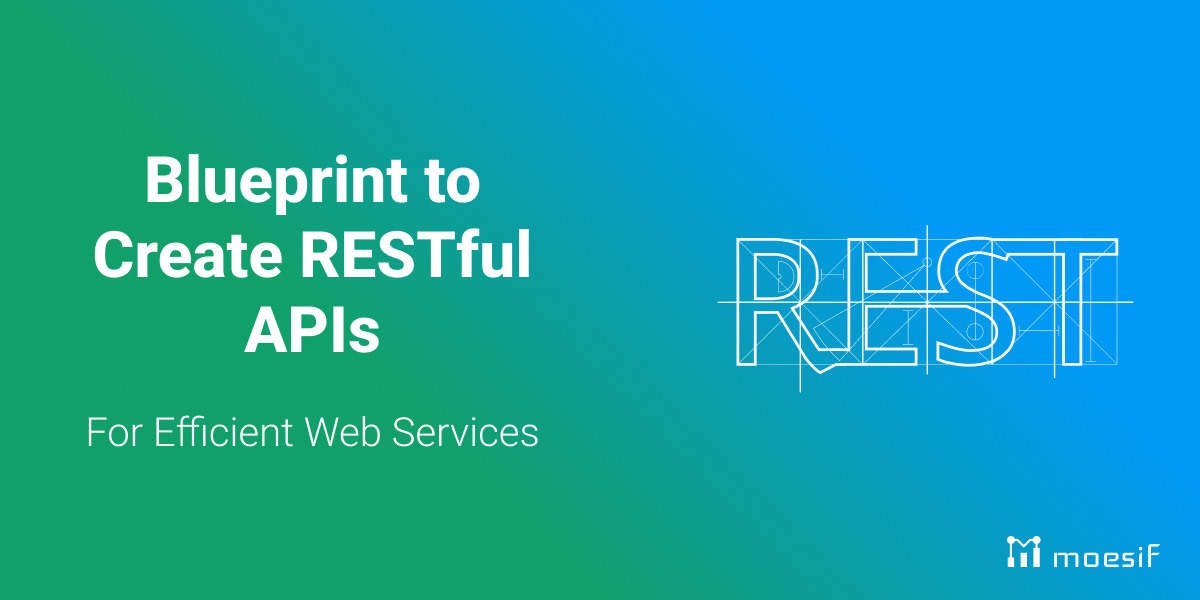
Creating RESTful APIs is essential for efficient web service development. With this article, you’ll learn to create restful apis using Node.js and Express by following a concise, step-by-step approach designed for real-world application. From server setup to endpoint creation and securing data, we’ve got you covered. This comprehensive guide will ensure you grasp the fundamentals while also diving into the more nuanced aspects of RESTful API development. You’ll gain insights into best practices for designing your API for maximum scalability and performance, and learn how to handle common challenges that arise during development. Whether you’re a novice eager to get started or an experienced developer looking to refine your skills, this article will provide valuable knowledge to enhance your web service projects.
Understanding REST Architecture
Diving into the subject matter, the REST architecture needs to be understood first. As an architectural style for building web services, REST promotes a clear separation between the client and the server, facilitating the independent progression and scaling of each component. This architectural design not only enhances scalability but also boosts reliability and predictability, thanks to each client-server interaction being stateless. That is to say, each request is self-contained with all the required details for its execution.
Beyond the client-server dichotomy, a uniform interface is another key aspect of REST. By abstracting the client from the server, it simplifies interactions and makes the system independently evolvable. A REST API, fundamentally, is a collection of standard protocols and guidelines that establish the framework for web services, using universal HTTP methods for interaction.
At the core of REST architecture lie six principles:
- Uniform interface
- Client-server architecture
- Statelessness
- Cacheability
- Layered system
- Optional code on demand
Each principle is instrumental in the design of REST APIs for efficient, scalable systems. We’ll explore these principles in greater depth, along with their practical applications, in the upcoming segments.
Crafting Your First REST API with Node.js and Express
As we step into the domain of REST API development, Node.js and Express stand out as the prime frameworks for developing straightforward REST API endpoints. From setting up the server.js file as the starting point to ensuring that the Node.js server is actively running for accessible API endpoints, the journey of building a REST API is an engaging endeavor.
The initial phase of this process involves establishing the appropriate environment. This initial setup is akin to laying the foundation for a building, providing a stable base on which to construct our API.
Step 1: Setting Up the Development Environment
The initial phase of this process involves establishing the appropriate environment. This initial setup is akin to laying the foundation for a building, providing a stable base on which to construct our API. Before diving into the coding aspect, it’s essential to prepare your development environment. Here’s a step-by-step guide to get you started:
Install Node.js and npm
Ensure Node.js and npm (Node Package Manager) are installed on your system. Node.js serves as the runtime environment, while npm is the package manager that allows you to install various libraries, including Express.
Create a New Project
Open your terminal or command prompt, navigate to the folder where you want to create your project, and run:
mkdir my-rest-api
cd my-rest-api
Initialize your Project
Initialize a Node.js Project To create a package.json
file which holds metadata about the project and its dependencies, run:
npm init -y
This command creates a package.json
file with default values.
Install Express
Inside your project directory, install Express using npm by running:
npm install express
This command adds Express to your project’s dependencies, allowing you to start building your REST API.
Step 2: Creating a Simple Server with Express
With the environment set up, let’s create a simple server using Express. This involves writing a small amount of code in a file, traditionally named server.js
, to start your server and listen for requests.
Create server.js
In your project directory, create a file named server.js
.
Write Server Code
Open server.js
in your text editor and add the following code:
// Import express
const express = require('express');
// Create an instance of express
const app = express();
// Define a port number
const port = 3000;
// Define a route for GET requests to '/'
app.get('/', (req, res) => {
res.send('Hello World! This is my first REST API.');
});
// Start the server
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
This code snippet does the following:
- Imports the Express library.
- Initializes an Express application.
- Sets up a basic route (
'/'
) that responds with a message when accessed via a GET request. - Starts the server on a specified port (3000 in this example), logging a message to the console once it’s running.
Run Your Server
To start your server, go back to your terminal and run:
node server.js
You should see a message indicating that the server is running. Open a web browser and navigate to http://localhost:3000
. You will be greeted with the message, “Hello World! This is my first REST API.”
Nice, you’ve just set up your development environment and created a simple server with Express to serve your first REST API endpoint! This forms the foundation upon which you can build more complex APIs, adding more routes and functionality as needed.
Defining Endpoints and HTTP Methods
Now that the groundwork has been laid, we can commence the building process. And in REST API development, building starts with defining endpoints and HTTP methods, including handling HTTP requests. To build a REST API, it’s essential to understand the standard HTTP methods - GET, PUT, POST, and DELETE - as these are the tools we use to define endpoints for CRUD (Create, Read, Update, Delete) operations, including handling delete requests. These methods, when combined with clear and intuitive endpoints, provide a structured interface that clients can use to manage information intuitively.
Creating a REST API involves more than just setting up a server; it requires defining specific paths (endpoints) and how they respond to different HTTP requests. This is crucial for performing CRUD operations. Let’s dive into how we can define these operations using HTTP methods like GET, POST, PUT, and DELETE, and set up a structured request and response system.
GET Request - Reading Data
Retrieve All Items
Let’s say we want to retrieve a list of items. We use a GET request for reading data.
app.get('/items', (req, res) => {
// Assuming 'items' is our data array
res.json(items); // Send all items as response
});
POST Request - Creating Data
Add a New Item
To create a new item, we use a POST request. This involves sending data (in the body of the request) from the client to the server.
// Middleware to parse request body
app.use(express.json());
app.post('/items', (req, res) => {
const newItem = req.body; // Data sent from the client
items.push(newItem); // Add item to our data array
res.status(201).send('Item added.');
});
PUT Request - Updating Data
Update an Existing Item
Updating data can be accomplished with a PUT request, specifying the item’s ID in the endpoint.
app.put('/items/:id', (req, res) => {
const id = req.params.id; // Get the item ID from the URL
const updatedItem = req.body; // Data for updating the item
// Logic to find and update the item by ID
const index = items.findIndex(item => item.id === id);
if (index !== -1) {
items[index] = updatedItem;
res.send('Item updated.');
} else {
res.status(404).send('Item not found.');
}
});
DELETE Request - Deleting Data
Remove an Item
For deleting an item, a DELETE request is used, also specifying the item’s ID.
app.delete('/items/:id', (req, res) => {
const id = req.params.id; // Get the item ID from the URL
// Logic to find and remove the item by ID
const index = items.findIndex(item => item.id === id);
if (index !== -1) {
items.splice(index, 1);
res.send('Item deleted.');
} else {
res.status(404).send('Item not found.');
}
});
By defining endpoints and handling HTTP methods appropriately, we lay the foundation for a robust REST API that allows clients to perform CRUD operations intuitively. This setup not only makes our API more structured but also ensures that it can handle complex data manipulation and interaction seamlessly.
Structuring the Request Body and Response Payload
In a REST API, both the request from the client and the response from the server typically use JSON (JavaScript Object Notation) for the body’s structure. This format is easy to read and write for humans and easy for machines to parse and generate.
- Request Body: When clients make POST or PUT requests, they send a JSON object in the request body, which contains the data to be created or updated.
- Response Payload: The server responds with a JSON object. This could be the created or updated data, a confirmation message, or, in the case of GET requests, an array of objects.
Using JSON ensures consistency in the way data is sent and received, making the API more intuitive to use. Furthermore, setting appropriate status codes (like 200 for success, 201 for created, 404 for not found) in the response helps the client understand the outcome of their request.
Preventing processing delays and potential errors can be achieved by evading unnecessary data and prudently utilizing nested data structures. This involves careful consideration of the data that is essential for each API call, ensuring that each JSON object sent in a request or response contains only what is necessary for that particular interaction. Avoiding overly complex or deeply nested structures helps to maintain clarity and aids in the processing speed of the API.
It’s important to establish a schema for the request and response data. This schema acts as a contract that defines the format, type, and constraints of the data elements within the API’s exchange. Utilizing schemas not only helps in validating the data but also serves as documentation for the expected data model, which can be extremely beneficial for both the API developers and consumers.
In addition to the structure, the way data is handled in the request body and response payload can significantly impact the performance and usability of the API. For example, pagination in the response payload can help manage large datasets by breaking them down into smaller, more manageable chunks. This improves the client’s ability to consume and process the data, as well as the efficiency of data transmission over the network.
Overall, thoughtful structuring of the request body and response payload is a critical aspect of RESTful API design that contributes to the creation of robust, scalable, and user-friendly APIs.
Designing Intuitive and Scalable REST APIs
Much like any design process, API design aims at developing something that is not only operational but also intuitive and capable of scaling. Horizontal scaling, for instance, is a preferable approach over vertical scaling for maintaining scalable and high-performing APIs.
Incorporating cache layers in HTTP and along the API request processing pipeline significantly contributes to sustaining API performance.
Data Handling in RESTful APIs
Data forms the core of APIs, and its efficient management is critical to the success of a RESTful API. JSON (JavaScript Object Notation) is one of the standardized data formats commonly used when interacting with RESTful APIs.
Along with data modeling, which is essential for identifying the structure of data and its interrelationships, JSON enables efficient operations in RESTful APIs.
Determine Resource Representations
Within the domain of REST API, the representation of resources is of paramount importance. Using plural nouns to represent resources in REST API endpoints makes the API more intuitive for those interacting with it. These endpoints, or URLs, represent resources in a web service, allowing for actions to be performed on these resources like creating, reading, updating, and deleting.
By meticulously structuring data, defining clear resource representations, and managing data transfer formats, developers lay the groundwork for powerful web services. It’s a process that demands attention to detail and a deep understanding of web standards, but the rewards—scalable, efficient, and user-friendly APIs—are well worth the effort.
Managing Data Transfer and Formats
Managing data transfer and choosing the right data format is as important as determining resource representations. The Last-Modified header, for instance, is used in content negotiation to indicate when the associated resource last changed, which is crucial for data transfer management.
Robust Error Handling Strategies
The foundation of dependable API development lies in robust error management. Implementing proper HTTP status codes and detailed error messages helps engineers quickly identify problems. Moreover, mapping all exceptions in an error payload that describes the origin of the error and provides guidance on how to address it offers clearer feedback during unexpected situations.
Securing Your RESTful API
Security holds utmost importance in every digital undertaking, including API development. Implementing authentication and authorization protocols like OAuth 2.0 is crucial for the security of your RESTful API. Additional security measures such as SSL/TLS encryption and fine-grained access control complement API key usage to protect sensitive information.
Enhancing Performance with Caching Techniques
To boost API performance, caching serves as an influential tool. Using data caching solutions like Redis and apicache improves the overall experience, serves often requested resources more quickly, and reduces querying from the database.
Cache-Control headers are used to manage the duration and location of cached responses, including their validation before use.
Testing Tools and Practices for REST APIs
API development is incomplete without the integral process of testing. Automated testing frameworks like Rspec, API Fortress, and Postman can enhance testing procedures, allowing for structured, extendable, reusable, and maintainable tests.
Prior to testing REST APIs, understanding API requirements, including query parameter usage, is imperative for proper test data and verification method preparation.
Documenting Your API for Maximum Usability
Documentation, being the initial interaction point for consumers with an API, aids developers in using the API effectively. Clear and thorough API documentation is crucial for understanding functionalities, endpoints, request/response formats, authentication, rate limits, and error handling. Including practical code examples within the documentation enhances developers’ understanding of how to implement the API.
Optimizing REST APIs for Third-Party Apps
The optimization of APIs for third-party apps focuses on facilitating effortless integration and updates. API versioning is essential for allowing service providers to introduce changes without disrupting existing clients. Optimizing API architecture for modularity helps manage complexity and isolates issues, facilitating the integration of superfluous services.
Summary
From understanding the core principles of REST architecture to the intricacies of crafting, testing, and optimizing REST APIs, we’ve embarked on a comprehensive journey through the world of RESTful APIs. As digital transformation continues to shape our world, the knowledge and skills shared in this blog post will empower you to contribute to this evolution.
Empower your API management with Moesif’s cutting-edge governance and monetization features. Govern user access and enforce quotas efficiently, while unlocking new revenue streams through flexible, usage-based billing models. Seamless integration ensures your API’s security and financial growth. Start revolutionizing your API strategy today by signing up for Moesif’s free trial, and take the first step towards optimized control and monetization of your digital services.