API Resources for Java Developers
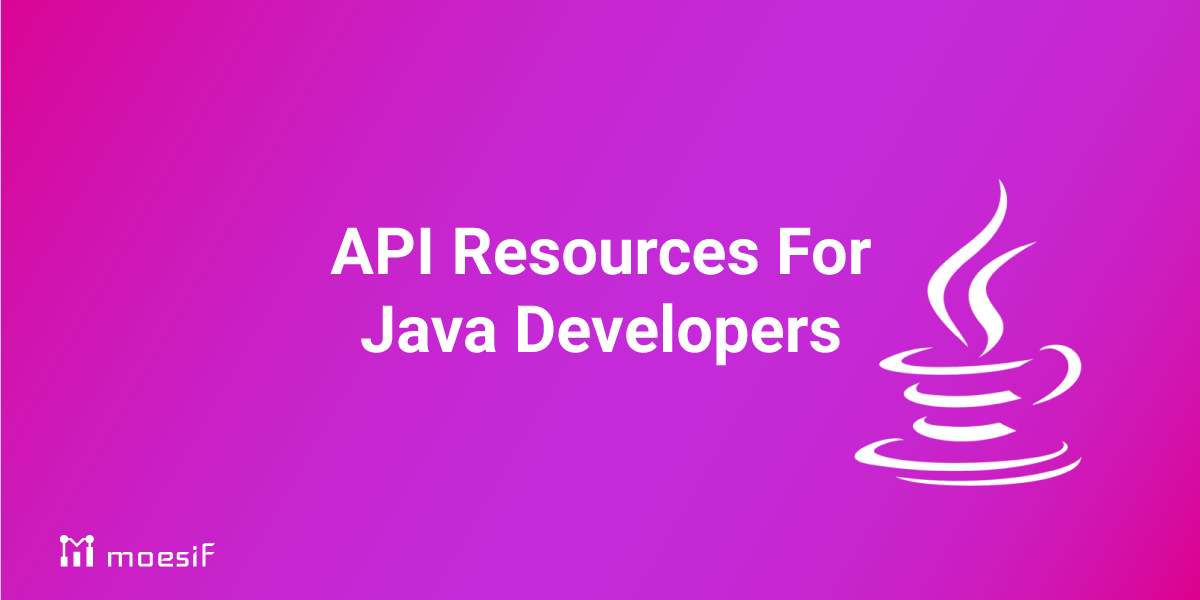
Java still remains one of the most popular programming languages and is often the platform of choice for building enterprise APIs. Java has come a long way from your grandfather’s J2EE powering old enterprise applications running on Tomcat and Java Servlet.
Modern Java frameworks from Spring to Play Framework now support non blocking IO along with HTTP servers like Netty. Java 8 (and 9) has brought many modern functional concepts into the language. Java is typically a little more resource heavy on memory relative to other languages, but you still can’t beat the flexibility and performance of it’s threading model along with a wide variety of lock free structures while threading is almost an after thought or non-existent in other languages.
Java and other JVM languages like Scala are very popular with big data applications at scale. Implementations like Akka provide a variety of concurrency models to work with on the JVM such as actor-baed concurrency. Popular big data tools from Kafka to Spark to Elasticsearch run on JVM.
Background on Java
- Extensive History and Information on Wikipedia
- Introduction of Java 8 really changed the landscape
- Understand the JVM - The engines that runs Java and many other languages such as Scala, Groovy, etc.
- Java 8 tutorial
- A series of video tutorial
Popular Frameworks
You can develop RESTful APIs without using frameworks, but use one of the popular web frameworks can make it a lot easier.
- Spring MVC - A complete HTTP oriented MVC framework managed by the Spring Framework and based in Servlets. It has an @RestController to implement REST based APIs.
- Spring Boot - Spring Boot makes it easy to create stand-alone, production-grade Spring based Applications that you can “just run”. It is an opinionated view of the Spring platform and third-party libraries so you can get started with minimum fuss. Basically it has a lot of default configuration.
- Grails Framework - A powerful Groovy-based web application framework for the JVM built on top of Spring Boot
- Apache Struts - Apache Struts is a free, open-source, MVC framework for creating elegant, modern Java web applications. It favors convention over configuration, is extensible using a plugin architecture, and ships with plugins to support REST, AJAX and JSON.
- Playframework - Play is based on a lightweight, stateless, web-friendly architecture. Built on Akka, Play provides predictable and minimal resource consumption (CPU, memory, threads) for highly-scalable applications.
Tutorials and Resources for Creating RESTFul APIs
These tutorials and resources are organized by major frameworks.
-
Spring MVC
-
Spring Boot
-
Grails Framework
-
Stuts
-
Playframework
- Making a REST API with play from Lightbend
- Multi-part series on Playframework and RESTful APIs
- [A tutorial with full source code](https://spr.com/building-a-simple-rest-api-with-scala-play-part-1
Tutorials for Creating GraphQL APIs
GraphQL is promoted as an alternative to RESTful API, you can read more about the background on GraphQL, and other types of APIs here.
- Getting started on GraphQL on Spring Boot
- GraphQL using Kotlin and Spring Boot
- Spring framework and GraphQL
- Tutorial from HowToGraphQL
- A tutorial using the Spark framework
Tutorials for Deployment
-
Heroku
-
AWS
-
Azure
-
Google App Engine
Useful Libraries
Maven is probably the most popular package manager for Java.
-
Documentation
- Spring Fox - The Springfox suite of java libraries are all about automating the generation of machine and human readable specifications for JSON APIs written using the spring family of projects. It evolved from swagger-springMVC.
- Swagger Play - swagger integration for Playframework.
- Spring REST docs - Document RESTful services by combining hand-written documentation with auto-generated snippets produced with Spring MVC Test.
- Enunciate - An enhancement engine for your java web service API.
-
Authentication
-
CORS
- cors-filter - A Java servlet filter implementation of server-side CORS
-
JSON
- Genson - Powerful and easy-to-use Java-to-JSON conversion library.
- Gson - Serializes objects to JSON and vice versa. Good performance with on-the-fly usage.
- HikariJSON - High-performance JSON parser, 2x faster than Jackson.
- jackson-modules-java8 - Set of Jackson modules for Java 8 datatypes and features.
- Jackson-datatype-money - Open-source Jackson module to support JSON serialization and deserialization of JavaMoney data types.
- Jackson - Similar to GSON, but offers performance gains if you need to instantiate the library more often.
- JSON-io - Convert Java to JSON. Convert JSON to Java. Pretty print JSON. Java JSON serializer.
- jsoniter - Fast and flexible library with iterator and lazy parsing API.
- LoganSquare - JSON parsing and serializing library based on Jackson’s streaming API. Outperforms GSON & Jackson’s library.
- Moshi - Modern JSON library, less opinionated and uses built-in types like List and Map.
- Yasson - Binding layer between classes and JSON documents similar to JAXB.
-
Databases
- FlexyPool - Brings metrics and failover strategies to the most common connection pooling solutions.
- Flyway - Simple database migration tool.
- HikariCP - High-performance JDBC connection pool.
- JDBI - Convenient abstraction of JDBC.
- Jedis - Small client for interaction with Redis, with methods for commands.
- jetcd - Client library for etcd.
- Jinq - Typesafe database queries via symbolic execution of Java 8 Lambdas (on top of JPA or jOOQ).
- jOOQ - Generates typesafe code based on SQL schema.
- MapDB - Embedded database engine that provides concurrent collections backed on disk or in off-heap memory.
- MariaDB4j - Launcher for MariaDB that requires no installation or external dependencies.
- Presto - Distributed SQL query engine for big data.
- Querydsl - Typesafe unified queries.
- Redisson - Allows for distributed and scalable data structures on top of a Redis server.
- requery - A modern, lightweight but powerful object mapping and SQL generator. Easily map to or create databases, or perform queries and updates from any Java-using platform.
- Speedment - Database access library that utilizes Java 8’s Stream API for querying.
- sql2o - Thin JDBC wrapper that simplifies database access and provides simple mapping of ResultSets to POJOs.
- Vibur DBCP - JDBC connection pool library with advanced performance monitoring capabilities.
-
ORMS
- Apache Cayenne - Provides a clean, static API for data access. Also includes a GUI Modeler for working with database mappings, and DB reverse engineering and generation.
- Ebean - Provides simple and fast data access.
- EclipseLink - Supports a number of persistence standards: JPA, JAXB, JCA and SDO.
- Hibernate - Robust and widely used, with an active community.
- MyBatis - Couples objects with stored procedures or SQL statements.
- SimpleFlatMapper - Simple database and CSV mapper.
-
Testing
- Apache JMeter - Functional testing and performance measurements.
- Arquillian - Integration and functional testing platform for Java EE containers.
- Citrus - Integration testing framework that focuses on both client- and server-side messaging.
- Gatling - Load testing tool designed for ease of use, maintainability and high performance.
- JUnit - Common testing framework.
-
REST
- Dropwizard - Opinionated framework for setting up modern web applications with Jetty, Jackson, Jersey and Metrics.
- Jersey - JAX-RS reference implementation.
- Microserver — A convenient, extensible microservices plugin system for Spring & Spring Boot. With more than 30 plugins and growing, it supports both micro-monolith and pure microservices styles.
- Rapidoid - A simple, secure and extremely fast framework consisting of an embedded HTTP server, GUI components and dependency injection.
- rest.li - Framework for building robust, scalable RESTful architectures using typesafe bindings and asynchronous, non-blocking IO with an end-to-end developer workflow that promotes clean practices, uniform interface design and consistent data modeling.
- RESTEasy - Fully certified and portable implementation of the JAX-RS specification.
- RestExpress - Thin wrapper on the JBoss Netty HTTP stack that provides scaling and performance.
- Restlet Framework - Pioneering framework with powerful routing and filtering capabilities, and a unified client and server API.
- Spark - Sinatra inspired framework.
- Crnk - Implementation of the JSON API specification to build resource-oriented REST endpoints with sorting, filtering, paging, linking, object graphs, type-safety, bulk updates, integrations and more.
-
REST Client
- restQL-core - Microservice query language that fetches information from multiple services.
- Retrofit - Typesafe REST client.
- Async Http Client - Asynchronous HTTP and WebSocket client library.
-
GraphQL
- graphql-java - Java implementation of GraphQL.
-
graphql-java-tools: A schema-first tool for graphql-java inspired by graphql-tools for JS
- graphql-java-type-generator: This library will autogenerate GraphQL types for usage in com.graphql-java:graphql-java
- graphql-java-annotations: Annotations-based syntax for GraphQL schema definition.
- spring-graphql-common: Spring Framework GraphQL Library
- graphql-emf: Support for EMF models and data
-
Rejoiner: Provides a uniform GraphQL schema on top of gRPC microservices by generating GraphQL types from Protobuf.
- rdbms-to-graphql: A Java CLI program that generates a GraphQL schema from a JDBC data source.
- graphql-java-servlet: Servlet that automatically exposes a schema dynamically built from GraphQL queries and mutations.
- graphql-spring-boot: GraphQL and GraphiQL Spring Framework Boot Starters
- graphql-jpa-spring-boot-starter: Spring Boot starter for GraphQL JPA; Expose JPA entities with GraphQL.
- Light Java GraphQL: A lightweight, fast microservices framework with all other cross-cutting concerns addressed that is ready to plug in GraphQL schema.
- Moqui GraphQL: An addon of Moqui framework to generate GraphQL Schema automatically.
- GORM GraphQL: An fully customizable addon for GORM (Grails Object Relational Model) to generate a GraphQL schema automatically.
- Vert.x GraphQL Utils - Vert.x route handler and Vert.x compatible interfaces to handle GraphQL queries in Vert.x applications.
- dropwizard-graphql - Dropwizard bundle for exposing a GraphQL endpoint.
-
spring-boot-starter-graphql - Spring Boot Starter for GraphQL.
- java-dataloader: A pure java 8 port of Facebook DataLoader
-
Utilities
- Apache Log4j 2 - Complete rewrite with a powerful plugin and configuration architecture.
- Dagger2 - Compile-time injection framework without reflection.
- JCTools - Concurrency tools currently missing from the JDK.
-
WebSockets
- Project Tyrus - JSR 356: Java API for WebSocket - Reference Implementation.
- Java-WebSocket - Barebones WebSocket client and server implementation written in 100% Java.
- Atmosphere - Realtime Client Server Framework for the JVM, supporting WebSockets with Cross-Browser Fallbacks.
- Webbit - Java event based WebSocket and HTTP server.